mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
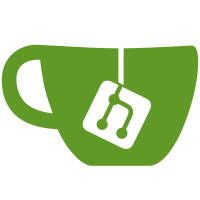
switchable between Tk (standard Tcl/Tk widget set) and Ttk (Tile). Initial default widget set is Tk. Now, toplevel widget classes are removed and defined as aliases. For example, "TkButton" is an alias of the "Tk::Button" class. Those aliases are replaced when switching default widget set. "Tk.default_widget_set=" is the method for switching default widget set. "Tk.default_widget_set = :Ttk" defines Ttk (Tile) widget set as default. It means that "TkButton" denotes "Tk::Tile::Button" class. And then, "TkButton.new" creates a Tk::Tile::Button widget. Of course, you can back to use standard Tk widgets as the default widget set by calling "Tk.default_widget_set = :Tk", whenever you want. Based on thie feature, you can use Ttk widget styling engine on your old Ruby/Tk application without modifying its source, if you don'tuse widget options unsupported on Ttk widgets (At first, call "Tk.default_widget_set = :Ttk", and next load and run your application). This is one step for supporting Tcl/Tk8.5 features. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@15618 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
102 lines
2.1 KiB
Ruby
102 lines
2.1 KiB
Ruby
#
|
|
# tkextlib/iwidgets/selectionbox.rb
|
|
# by Hidetoshi NAGAI (nagai@ai.kyutech.ac.jp)
|
|
#
|
|
|
|
require 'tk'
|
|
require 'tkextlib/iwidgets.rb'
|
|
|
|
module Tk
|
|
module Iwidgets
|
|
class Selectionbox < Tk::Itk::Widget
|
|
end
|
|
end
|
|
end
|
|
|
|
class Tk::Iwidgets::Selectionbox
|
|
TkCommandNames = ['::iwidgets::selectionbox'.freeze].freeze
|
|
WidgetClassName = 'Selectionbox'.freeze
|
|
WidgetClassNames[WidgetClassName] = self
|
|
|
|
def __boolval_optkeys
|
|
super() << 'itemson' << 'selectionon'
|
|
end
|
|
private :__boolval_optkeys
|
|
|
|
def __strval_optkeys
|
|
super() << 'itemslabel' << 'selectionlabel'
|
|
end
|
|
private :__strval_optkeys
|
|
|
|
def child_site
|
|
window(tk_call(@path, 'childsite'))
|
|
end
|
|
|
|
def clear_items
|
|
tk_call(@path, 'clear', 'items')
|
|
self
|
|
end
|
|
|
|
def clear_selection
|
|
tk_call(@path, 'clear', 'selection')
|
|
self
|
|
end
|
|
|
|
def get
|
|
tk_call(@path, 'get')
|
|
end
|
|
|
|
def insert_items(idx, *args)
|
|
tk_call(@path, 'insert', 'items', idx, *args)
|
|
end
|
|
|
|
def insert_selection(pos, text)
|
|
tk_call(@path, 'insert', 'selection', pos, text)
|
|
end
|
|
|
|
def select_item
|
|
tk_call(@path, 'selectitem')
|
|
self
|
|
end
|
|
|
|
# based on Tk::Listbox ( and TkTextWin )
|
|
def curselection
|
|
list(tk_send_without_enc('curselection'))
|
|
end
|
|
def delete(first, last=None)
|
|
tk_send_without_enc('delete', first, last)
|
|
self
|
|
end
|
|
def index(idx)
|
|
tk_send_without_enc('index', idx).to_i
|
|
end
|
|
def nearest(y)
|
|
tk_send_without_enc('nearest', y).to_i
|
|
end
|
|
def scan_mark(x, y)
|
|
tk_send_without_enc('scan', 'mark', x, y)
|
|
self
|
|
end
|
|
def scan_dragto(x, y)
|
|
tk_send_without_enc('scan', 'dragto', x, y)
|
|
self
|
|
end
|
|
def selection_anchor(index)
|
|
tk_send_without_enc('selection', 'anchor', index)
|
|
self
|
|
end
|
|
def selection_clear(first, last=None)
|
|
tk_send_without_enc('selection', 'clear', first, last)
|
|
self
|
|
end
|
|
def selection_includes(index)
|
|
bool(tk_send_without_enc('selection', 'includes', index))
|
|
end
|
|
def selection_set(first, last=None)
|
|
tk_send_without_enc('selection', 'set', first, last)
|
|
self
|
|
end
|
|
def size
|
|
tk_send_without_enc('size').to_i
|
|
end
|
|
end
|