mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
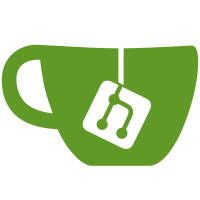
* Other ruby implementations use the spec/ruby directory. [Misc #13792] [ruby-core:82287] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@59979 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
49 lines
1.2 KiB
Ruby
49 lines
1.2 KiB
Ruby
# -*- encoding: binary -*-
|
|
module EncodingSpecs
|
|
class UndefinedConversionError
|
|
def self.exception
|
|
ec = Encoding::Converter.new('utf-8','ascii')
|
|
begin
|
|
ec.convert("\u{8765}")
|
|
rescue Encoding::UndefinedConversionError => e
|
|
e
|
|
end
|
|
end
|
|
end
|
|
|
|
class UndefinedConversionErrorIndirect
|
|
def self.exception
|
|
ec = Encoding::Converter.new("ISO-8859-1", "EUC-JP")
|
|
begin
|
|
ec.convert("\xA0")
|
|
rescue Encoding::UndefinedConversionError => e
|
|
e
|
|
end
|
|
end
|
|
end
|
|
|
|
class InvalidByteSequenceError
|
|
def self.exception
|
|
ec = Encoding::Converter.new("utf-8", "iso-8859-1")
|
|
begin
|
|
ec.convert("\xf1abcd")
|
|
rescue Encoding::InvalidByteSequenceError => e
|
|
# Return the exception object and the primitive_errinfo Array
|
|
[e, ec.primitive_errinfo]
|
|
end
|
|
end
|
|
end
|
|
|
|
class InvalidByteSequenceErrorIndirect
|
|
def self.exception
|
|
ec = Encoding::Converter.new("EUC-JP", "ISO-8859-1")
|
|
begin
|
|
ec.convert("abc\xA1\xFFdef")
|
|
rescue Encoding::InvalidByteSequenceError => e
|
|
# Return the exception object and the discarded bytes reported by
|
|
# #primitive_errinfo
|
|
[e, ec.primitive_errinfo]
|
|
end
|
|
end
|
|
end
|
|
end
|