mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
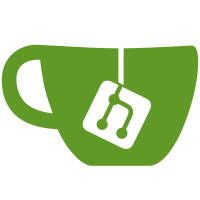
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@6237 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
142 lines
3.7 KiB
Ruby
142 lines
3.7 KiB
Ruby
#
|
|
# tk/event.rb - module for event
|
|
#
|
|
require 'tk'
|
|
|
|
module TkEvent
|
|
class Event < TkUtil::CallbackSubst
|
|
module TypeNum
|
|
KeyPress = 2
|
|
KeyRelease = 3
|
|
ButtonPress = 4
|
|
ButtonRelease = 5
|
|
MotionNotify = 6
|
|
EnterNotify = 7
|
|
LeaveNotify = 8
|
|
FocusIn = 9
|
|
FocusOut = 10
|
|
KeymapNotify = 11
|
|
Expose = 12
|
|
GraphicsExpose = 13
|
|
NoExpose = 14
|
|
VisibilityNotify = 15
|
|
CreateNotify = 16
|
|
DestroyNotify = 17
|
|
UnmapNotify = 18
|
|
MapNotify = 19
|
|
MapRequest = 20
|
|
ReparentNotify = 21
|
|
ConfigureNotify = 22
|
|
ConfigureRequest = 23
|
|
GravityNotify = 24
|
|
ResizeRequest = 25
|
|
CirculateNotify = 26
|
|
CirculateRequest = 27
|
|
PropertyNotify = 28
|
|
SelectionClear = 29
|
|
SelectionRequest = 30
|
|
SelectionNotify = 31
|
|
ColormapNotify = 32
|
|
ClientMessage = 33
|
|
MappingNotify = 34
|
|
end
|
|
|
|
# [ <'%' subst-key char>, <proc type char>, <instance var (accessor) name>]
|
|
key_tbl = [
|
|
[ ?#, ?n, :serial ],
|
|
[ ?a, ?s, :above ],
|
|
[ ?b, ?n, :num ],
|
|
[ ?c, ?n, :count ],
|
|
[ ?d, ?s, :detail ],
|
|
[ ?f, ?b, :focus ],
|
|
[ ?h, ?n, :height ],
|
|
[ ?i, ?s, :win_hex ],
|
|
[ ?k, ?n, :keycode ],
|
|
[ ?m, ?s, :mode ],
|
|
[ ?o, ?b, :override ],
|
|
[ ?p, ?s, :place ],
|
|
[ ?s, ?x, :state ],
|
|
[ ?t, ?n, :time ],
|
|
[ ?w, ?n, :width ],
|
|
[ ?x, ?n, :x ],
|
|
[ ?y, ?n, :y ],
|
|
[ ?A, ?s, :char ],
|
|
[ ?B, ?n, :borderwidth ],
|
|
[ ?D, ?n, :wheel_delta ],
|
|
[ ?E, ?b, :send_event ],
|
|
[ ?K, ?s, :keysym ],
|
|
[ ?N, ?n, :keysym_num ],
|
|
[ ?R, ?s, :rootwin_id ],
|
|
[ ?S, ?s, :subwindow ],
|
|
[ ?T, ?n, :type ],
|
|
[ ?W, ?w, :widget ],
|
|
[ ?X, ?n, :x_root ],
|
|
[ ?Y, ?n, :y_root ],
|
|
nil
|
|
]
|
|
|
|
# [ <proc type char>, <proc/method to convert tcl-str to ruby-obj>]
|
|
proc_tbl = [
|
|
[ ?n, TkComm.method(:num_or_str) ],
|
|
[ ?s, TkComm.method(:string) ],
|
|
[ ?b, TkComm.method(:bool) ],
|
|
[ ?w, TkComm.method(:window) ],
|
|
|
|
[ ?x, proc{|val|
|
|
begin
|
|
TkComm::number(val)
|
|
rescue ArgumentError
|
|
val
|
|
end
|
|
}
|
|
],
|
|
|
|
nil
|
|
]
|
|
|
|
# setup tables to be used by scan_args, _get_subst_key, _get_all_subst_keys
|
|
#
|
|
# _get_subst_key() and _get_all_subst_keys() generates key-string
|
|
# which describe how to convert callback arguments to ruby objects.
|
|
# When binding parameters are given, use _get_subst_key().
|
|
# But when no parameters are given, use _get_all_subst_keys() to
|
|
# create a Event class object as a callback parameter.
|
|
#
|
|
# scan_args() is used when doing callback. It convert arguments
|
|
# ( which are Tcl strings ) to ruby objects based on the key string
|
|
# that is generated by _get_subst_key() or _get_all_subst_keys().
|
|
#
|
|
_setup_subst_table(key_tbl, proc_tbl);
|
|
end
|
|
|
|
def install_bind(cmd, *args)
|
|
if args.compact.size > 0
|
|
args = args.join(' ')
|
|
keys = Event._get_subst_key(args)
|
|
|
|
if cmd.kind_of?(String)
|
|
id = cmd
|
|
elsif cmd.kind_of?(TkCallbackEntry)
|
|
id = install_cmd(cmd)
|
|
else
|
|
id = install_cmd(proc{|*arg|
|
|
TkUtil.eval_cmd(cmd, *Event.scan_args(keys, arg))
|
|
})
|
|
end
|
|
id + ' ' + args
|
|
else
|
|
keys, args = Event._get_all_subst_keys
|
|
|
|
if cmd.kind_of?(String)
|
|
id = cmd
|
|
elsif cmd.kind_of?(TkCallbackEntry)
|
|
id = install_cmd(cmd)
|
|
else
|
|
id = install_cmd(proc{|*arg|
|
|
TkUtil.eval_cmd(cmd, Event.new(*Event.scan_args(keys, arg)))
|
|
})
|
|
end
|
|
id + ' ' + args
|
|
end
|
|
end
|
|
end
|