mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
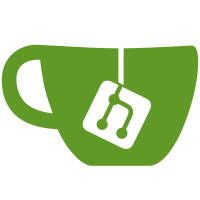
deflate-encoded response bodies. [Feature #6942] * lib/net/http/generic_request.rb: Automatically accept gzip and deflate content-encoding for requests. [Feature #6494] * lib/net/http/request.rb: Updated documentation for #6494. * lib/net/http.rb: Updated documentation for #6492 and #6494, removed Content-Encoding handling now present in Net::HTTPResponse. * test/net/http/test_httpresponse.rb: Tests for #6492 * test/net/http/test_http_request.rb: Tests for #6494 * test/open-uri/test_open-uri.rb (test_content_encoding): Updated test for automatic content-encoding handling. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@36473 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
57 lines
1.2 KiB
Ruby
57 lines
1.2 KiB
Ruby
require 'net/http'
|
|
require 'test/unit'
|
|
require 'stringio'
|
|
|
|
class HTTPRequestTest < Test::Unit::TestCase
|
|
|
|
def test_initialize_GET
|
|
req = Net::HTTP::Get.new '/'
|
|
|
|
assert_equal 'GET', req.method
|
|
refute req.request_body_permitted?
|
|
assert req.response_body_permitted?
|
|
|
|
expected = {
|
|
'accept' => %w[*/*],
|
|
'user-agent' => %w[Ruby],
|
|
}
|
|
|
|
expected['accept-encoding'] = %w[gzip;q=1.0,deflate;q=0.6,identity;q=0.3] if
|
|
Net::HTTP::HAVE_ZLIB
|
|
|
|
assert_equal expected, req.to_hash
|
|
end
|
|
|
|
def test_initialize_GET_range
|
|
req = Net::HTTP::Get.new '/', 'Range' => 'bytes=0-9'
|
|
|
|
assert_equal 'GET', req.method
|
|
refute req.request_body_permitted?
|
|
assert req.response_body_permitted?
|
|
|
|
expected = {
|
|
'accept' => %w[*/*],
|
|
'user-agent' => %w[Ruby],
|
|
'range' => %w[bytes=0-9],
|
|
}
|
|
|
|
assert_equal expected, req.to_hash
|
|
end
|
|
|
|
def test_initialize_HEAD
|
|
req = Net::HTTP::Head.new '/'
|
|
|
|
assert_equal 'HEAD', req.method
|
|
refute req.request_body_permitted?
|
|
refute req.response_body_permitted?
|
|
|
|
expected = {
|
|
'accept' => %w[*/*],
|
|
'user-agent' => %w[Ruby],
|
|
}
|
|
|
|
assert_equal expected, req.to_hash
|
|
end
|
|
|
|
end
|
|
|