mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
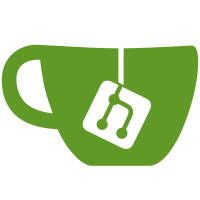
https://gist.github.com/ko1/72c03695e81a54d40649f29d0c421f26 git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@66412 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
57 lines
1.8 KiB
Ruby
57 lines
1.8 KiB
Ruby
require_relative '../../spec_helper'
|
|
require_relative 'fixtures/classes'
|
|
|
|
describe "IO#initialize" do
|
|
before :each do
|
|
@name = tmp("io_initialize.txt")
|
|
@io = new_io @name
|
|
@fd = @io.fileno
|
|
end
|
|
|
|
after :each do
|
|
@io.close if @io
|
|
rm_r @name
|
|
end
|
|
|
|
# File descriptor numbers are not predictable in multi-threaded code;
|
|
# MJIT will be opening/closing files the background
|
|
without_feature :mjit do
|
|
it "reassociates the IO instance with the new descriptor when passed a Fixnum" do
|
|
fd = new_fd @name, "r:utf-8"
|
|
@io.send :initialize, fd, 'r'
|
|
@io.fileno.should == fd
|
|
# initialize has closed the old descriptor
|
|
lambda { IO.for_fd(@fd).close }.should raise_error(Errno::EBADF)
|
|
end
|
|
|
|
it "calls #to_int to coerce the object passed as an fd" do
|
|
obj = mock('fileno')
|
|
fd = new_fd @name, "r:utf-8"
|
|
obj.should_receive(:to_int).and_return(fd)
|
|
@io.send :initialize, obj, 'r'
|
|
@io.fileno.should == fd
|
|
# initialize has closed the old descriptor
|
|
lambda { IO.for_fd(@fd).close }.should raise_error(Errno::EBADF)
|
|
end
|
|
end
|
|
|
|
it "raises a TypeError when passed an IO" do
|
|
lambda { @io.send :initialize, STDOUT, 'w' }.should raise_error(TypeError)
|
|
end
|
|
|
|
it "raises a TypeError when passed nil" do
|
|
lambda { @io.send :initialize, nil, 'w' }.should raise_error(TypeError)
|
|
end
|
|
|
|
it "raises a TypeError when passed a String" do
|
|
lambda { @io.send :initialize, "4", 'w' }.should raise_error(TypeError)
|
|
end
|
|
|
|
it "raises IOError on closed stream" do
|
|
lambda { @io.send :initialize, IOSpecs.closed_io.fileno }.should raise_error(IOError)
|
|
end
|
|
|
|
it "raises an Errno::EBADF when given an invalid file descriptor" do
|
|
lambda { @io.send :initialize, -1, 'w' }.should raise_error(Errno::EBADF)
|
|
end
|
|
end
|