mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
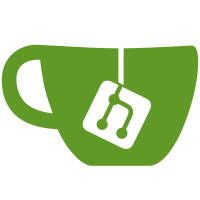
This commit reintroduces finer-grained constant cache invalidation.
After 8008fb7
got merged, it was causing issues on token-threaded
builds (such as on Windows).
The issue was that when you're iterating through instruction sequences
and using the translator functions to get back the instruction structs,
you're either using `rb_vm_insn_null_translator` or
`rb_vm_insn_addr2insn2` depending if it's a direct-threading build.
`rb_vm_insn_addr2insn2` does some normalization to always return to
you the non-trace version of whatever instruction you're looking at.
`rb_vm_insn_null_translator` does not do that normalization.
This means that when you're looping through the instructions if you're
trying to do an opcode comparison, it can change depending on the type
of threading that you're using. This can be very confusing. So, this
commit creates a new translator function
`rb_vm_insn_normalizing_translator` to always return the non-trace
version so that opcode comparisons don't have to worry about different
configurations.
[Feature #18589]
73 lines
1.5 KiB
Ruby
73 lines
1.5 KiB
Ruby
# frozen_string_literal: false
|
|
require 'test/unit'
|
|
|
|
class TestRubyVM < Test::Unit::TestCase
|
|
def test_stat
|
|
assert_kind_of Hash, RubyVM.stat
|
|
assert_kind_of Integer, RubyVM.stat[:class_serial]
|
|
|
|
RubyVM.stat(stat = {})
|
|
assert_not_empty stat
|
|
assert_equal stat[:class_serial], RubyVM.stat(:class_serial)
|
|
end
|
|
|
|
def test_stat_unknown
|
|
assert_raise(ArgumentError){ RubyVM.stat(:unknown) }
|
|
assert_raise_with_message(ArgumentError, /\u{30eb 30d3 30fc}/) {RubyVM.stat(:"\u{30eb 30d3 30fc}")}
|
|
end
|
|
|
|
def parse_and_compile
|
|
script = <<~RUBY
|
|
_a = 1
|
|
def foo
|
|
_b = 2
|
|
end
|
|
1.times{
|
|
_c = 3
|
|
}
|
|
RUBY
|
|
|
|
ast = RubyVM::AbstractSyntaxTree.parse(script)
|
|
iseq = RubyVM::InstructionSequence.compile(script)
|
|
|
|
[ast, iseq]
|
|
end
|
|
|
|
def test_keep_script_lines
|
|
pend if ENV['RUBY_ISEQ_DUMP_DEBUG'] # TODO
|
|
|
|
prev_conf = RubyVM.keep_script_lines
|
|
|
|
# keep
|
|
RubyVM.keep_script_lines = true
|
|
|
|
ast, iseq = *parse_and_compile
|
|
|
|
lines = ast.script_lines
|
|
assert_equal Array, lines.class
|
|
|
|
lines = iseq.script_lines
|
|
assert_equal Array, lines.class
|
|
iseq.each_child{|child|
|
|
assert_equal lines, child.script_lines
|
|
}
|
|
assert lines.frozen?
|
|
|
|
# don't keep
|
|
RubyVM.keep_script_lines = false
|
|
|
|
ast, iseq = *parse_and_compile
|
|
|
|
lines = ast.script_lines
|
|
assert_equal nil, lines
|
|
|
|
lines = iseq.script_lines
|
|
assert_equal nil, lines
|
|
iseq.each_child{|child|
|
|
assert_equal lines, child.script_lines
|
|
}
|
|
|
|
ensure
|
|
RubyVM.keep_script_lines = prev_conf
|
|
end
|
|
end
|