mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
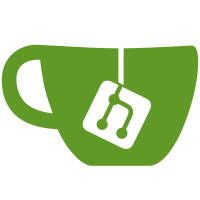
Integer overflow for unsigned types are fully defined in C. They are not always problematic (but not always OK). These functions in this changeset intentionally utilizes that behaviour. Blacklist from UBSAN checks for better output. See also: https://travis-ci.org/ruby/ruby/jobs/451624829 git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@65589 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
48 lines
1.3 KiB
C
48 lines
1.3 KiB
C
#ifndef SIPHASH_H
|
|
#define SIPHASH_H 1
|
|
#include <stdlib.h>
|
|
#ifdef HAVE_STDINT_H
|
|
#include <stdint.h>
|
|
#endif
|
|
#ifdef HAVE_INTTYPES_H
|
|
#include <inttypes.h>
|
|
#endif
|
|
|
|
#ifndef HAVE_UINT64_T
|
|
typedef struct {
|
|
uint32_t u32[2];
|
|
} sip_uint64_t;
|
|
#define uint64_t sip_uint64_t
|
|
#else
|
|
typedef uint64_t sip_uint64_t;
|
|
#endif
|
|
|
|
typedef struct {
|
|
int c;
|
|
int d;
|
|
uint64_t v[4];
|
|
uint8_t buf[sizeof(uint64_t)];
|
|
uint8_t buflen;
|
|
uint8_t msglen_byte;
|
|
} sip_state;
|
|
|
|
typedef struct sip_interface_st sip_interface;
|
|
|
|
typedef struct {
|
|
sip_state state[1];
|
|
const sip_interface *methods;
|
|
} sip_hash;
|
|
|
|
sip_hash *sip_hash_new(const uint8_t key[16], int c, int d);
|
|
sip_hash *sip_hash_init(sip_hash *h, const uint8_t key[16], int c, int d);
|
|
int sip_hash_update(sip_hash *h, const uint8_t *data, size_t len);
|
|
int sip_hash_final(sip_hash *h, uint8_t **digest, size_t *len);
|
|
int sip_hash_final_integer(sip_hash *h, uint64_t *digest);
|
|
int sip_hash_digest(sip_hash *h, const uint8_t *data, size_t data_len, uint8_t **digest, size_t *digest_len);
|
|
int sip_hash_digest_integer(sip_hash *h, const uint8_t *data, size_t data_len, uint64_t *digest);
|
|
void sip_hash_free(sip_hash *h);
|
|
void sip_hash_dump(sip_hash *h);
|
|
|
|
NO_SANITIZE("unsigned-integer-overflow", uint64_t sip_hash13(const uint8_t key[16], const uint8_t *data, size_t len));
|
|
|
|
#endif
|