mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
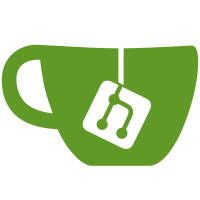
* ext/openssl/ossl_x509cert.c (ossl_x509_verify): X509_verify() family may put errors on 0 return (0 means verification failure). Clear OpenSSL error queue before return to Ruby. Since the queue is thread global, remaining errors in the queue can cause an unexpected error in the next OpenSSL operation. [ruby-core:48284] [Bug #7215] * ext/openssl/ossl_x509crl.c (ossl_x509crl_verify): ditto. * ext/openssl/ossl_x509req.c (ossl_x509req_verify): ditto. * ext/openssl/ossl_x509store.c (ossl_x509stctx_verify): ditto. * ext/openssl/ossl_pkey_dh.c (dh_generate): clear the OpenSSL error queue before re-raising exception. * ext/openssl/ossl_pkey_dsa.c (dsa_generate): ditto. * ext/openssl/ossl_pkey_rsa.c (rsa_generate): ditto. * ext/openssl/ossl_ssl.c (ossl_start_ssl): ditto. * test/openssl: check that OpenSSL.errors is empty every time after running a test case. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@55051 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
48 lines
1.6 KiB
Ruby
48 lines
1.6 KiB
Ruby
# frozen_string_literal: false
|
|
require_relative "utils"
|
|
|
|
if defined?(OpenSSL::TestUtils)
|
|
|
|
class OpenSSL::TestOCSP < OpenSSL::TestCase
|
|
def setup
|
|
ca_subj = OpenSSL::X509::Name.parse("/DC=org/DC=ruby-lang/CN=TestCA")
|
|
ca_key = OpenSSL::TestUtils::TEST_KEY_RSA1024
|
|
ca_serial = 0xabcabcabcabc
|
|
|
|
subj = OpenSSL::X509::Name.parse("/DC=org/DC=ruby-lang/CN=TestCert")
|
|
@key = OpenSSL::TestUtils::TEST_KEY_RSA1024
|
|
serial = 0xabcabcabcabd
|
|
|
|
now = Time.at(Time.now.to_i) # suppress usec
|
|
dgst = OpenSSL::Digest::SHA1.new
|
|
|
|
@ca_cert = OpenSSL::TestUtils.issue_cert(
|
|
ca_subj, ca_key, ca_serial, now, now+3600, [], nil, nil, dgst)
|
|
@cert = OpenSSL::TestUtils.issue_cert(
|
|
subj, @key, serial, now, now+3600, [], @ca_cert, nil, dgst)
|
|
end
|
|
|
|
def test_new_certificate_id
|
|
cid = OpenSSL::OCSP::CertificateId.new(@cert, @ca_cert)
|
|
assert_kind_of OpenSSL::OCSP::CertificateId, cid
|
|
assert_equal @cert.serial, cid.serial
|
|
end
|
|
|
|
def test_new_certificate_id_with_digest
|
|
cid = OpenSSL::OCSP::CertificateId.new(@cert, @ca_cert, OpenSSL::Digest::SHA256.new)
|
|
assert_kind_of OpenSSL::OCSP::CertificateId, cid
|
|
assert_equal @cert.serial, cid.serial
|
|
end if defined?(OpenSSL::Digest::SHA256)
|
|
|
|
def test_new_ocsp_request
|
|
request = OpenSSL::OCSP::Request.new
|
|
cid = OpenSSL::OCSP::CertificateId.new(@cert, @ca_cert, OpenSSL::Digest::SHA1.new)
|
|
request.add_certid(cid)
|
|
request.sign(@cert, @key, [@cert])
|
|
assert_kind_of OpenSSL::OCSP::Request, request
|
|
# in current implementation not same instance of certificate id, but should contain same data
|
|
assert_equal cid.serial, request.certid.first.serial
|
|
end
|
|
end
|
|
|
|
end
|