mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
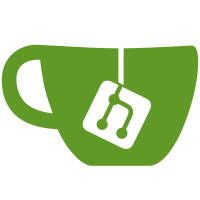
To perform a regular method call, the VM needs two structs, `rb_call_info` and `rb_call_cache`. At the moment, we allocate these two structures in separate buffers. In the worst case, the CPU needs to read 4 cache lines to complete a method call. Putting the two structures together reduces the maximum number of cache line reads to 2. Combining the structures also saves 8 bytes per call site as the current layout uses separate two pointers for the call info and the call cache. This saves about 2 MiB on Discourse. This change improves the Optcarrot benchmark at least 3%. For more details, see attached bugs.ruby-lang.org ticket. Complications: - A new instruction attribute `comptime_sp_inc` is introduced to calculate SP increase at compile time without using call caches. At compile time, a `TS_CALLDATA` operand points to a call info struct, but at runtime, the same operand points to a call data struct. Instruction that explicitly define `sp_inc` also need to define `comptime_sp_inc`. - MJIT code for copying call cache becomes slightly more complicated. - This changes the bytecode format, which might break existing tools. [Misc #16258]
59 lines
1.5 KiB
Ruby
59 lines
1.5 KiB
Ruby
#! /your/favourite/path/to/ruby
|
|
# -*- Ruby -*-
|
|
# -*- frozen_string_literal: true; -*-
|
|
# -*- warn_indent: true; -*-
|
|
#
|
|
# Copyright (c) 2017 Urabe, Shyouhei. All rights reserved.
|
|
#
|
|
# This file is a part of the programming language Ruby. Permission is hereby
|
|
# granted, to either redistribute and/or modify this file, provided that the
|
|
# conditions mentioned in the file COPYING are met. Consult the file for
|
|
# details.
|
|
|
|
require_relative 'c_expr'
|
|
|
|
class RubyVM::Attribute
|
|
include RubyVM::CEscape
|
|
attr_reader :insn, :key, :type, :expr
|
|
|
|
def initialize opts = {}
|
|
@insn = opts[:insn]
|
|
@key = opts[:name]
|
|
@expr = RubyVM::CExpr.new location: opts[:location], expr: opts[:expr]
|
|
@type = opts[:type]
|
|
@ope_decls = @insn.opes.map do |operand|
|
|
decl = operand[:decl]
|
|
if @key == 'comptime_sp_inc' && operand[:type] == 'CALL_DATA'
|
|
decl = decl.gsub('CALL_DATA', 'CALL_INFO').gsub('cd', 'ci')
|
|
end
|
|
decl
|
|
end
|
|
end
|
|
|
|
def name
|
|
as_tr_cpp "attr #{@key} @ #{@insn.name}"
|
|
end
|
|
|
|
def pretty_name
|
|
"attr #{type} #{key} @ #{insn.pretty_name}"
|
|
end
|
|
|
|
def declaration
|
|
if @ope_decls.empty?
|
|
argv = "void"
|
|
else
|
|
argv = @ope_decls.join(', ')
|
|
end
|
|
sprintf '%s %s(%s)', @type, name, argv
|
|
end
|
|
|
|
def definition
|
|
if @ope_decls.empty?
|
|
argv = "void"
|
|
else
|
|
argv = @ope_decls.map {|decl| "MAYBE_UNUSED(#{decl})" }.join(",\n ")
|
|
argv = "\n #{argv}\n" if @ope_decls.size > 1
|
|
end
|
|
sprintf "%s\n%s(%s)", @type, name, argv
|
|
end
|
|
end
|