mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
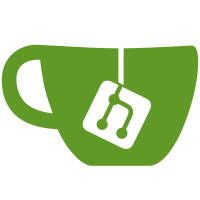
When you change this to true, you may need to add more tests. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@53141 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
53 lines
1 KiB
Ruby
53 lines
1 KiB
Ruby
# frozen_string_literal: false
|
|
require_relative 'helper'
|
|
require 'tempfile'
|
|
|
|
module DTrace
|
|
class TestLoad < TestCase
|
|
def setup
|
|
super
|
|
@rbfile = Tempfile.new(['omg', 'rb'])
|
|
@rbfile.write 'x = 10'
|
|
end
|
|
|
|
def teardown
|
|
super
|
|
@rbfile.close(true) if @rbfile
|
|
end
|
|
|
|
def test_load_entry
|
|
probe = <<-eoprobe
|
|
ruby$target:::load-entry
|
|
{
|
|
printf("%s %s %d\\n", copyinstr(arg0), copyinstr(arg1), arg2);
|
|
}
|
|
eoprobe
|
|
trap_probe(probe, program) { |dpath, rbpath, saw|
|
|
saw = saw.map(&:split).find_all { |loaded, _, _|
|
|
loaded == @rbfile.path
|
|
}
|
|
assert_equal 10, saw.length
|
|
}
|
|
end
|
|
|
|
def test_load_return
|
|
probe = <<-eoprobe
|
|
ruby$target:::load-return
|
|
{
|
|
printf("%s\\n", copyinstr(arg0));
|
|
}
|
|
eoprobe
|
|
trap_probe(probe, program) { |dpath, rbpath, saw|
|
|
saw = saw.map(&:split).find_all { |loaded, _, _|
|
|
loaded == @rbfile.path
|
|
}
|
|
assert_equal 10, saw.length
|
|
}
|
|
end
|
|
|
|
private
|
|
def program
|
|
"10.times { load '#{@rbfile.path}' }"
|
|
end
|
|
end
|
|
end if defined?(DTrace::TestCase)
|