mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
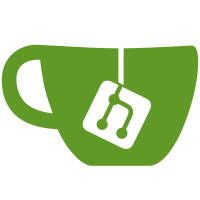
[ruby-trunk - Feature #6435] * lib/net/http/backward.rb: ditto. * lib/net/http/response.rb: ditto. * lib/net/http/exceptions.rb: ditto. * lib/net/http/responses.rb: ditto. * lib/net/http/generic_request.rb: ditto. * lib/net/http/header.rb: ditto. * lib/net/http/request.rb: ditto. * lib/net/http/proxy_delta.rb: ditto. * lib/net/http/requests.rb: ditto. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@35761 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
122 lines
2.9 KiB
Ruby
122 lines
2.9 KiB
Ruby
#
|
|
# HTTP/1.1 methods --- RFC2616
|
|
#
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
# See Net::HTTP for usage examples.
|
|
class Net::HTTP::Get < Net::HTTPRequest
|
|
METHOD = 'GET'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
# See Net::HTTP for usage examples.
|
|
class Net::HTTP::Head < Net::HTTPRequest
|
|
METHOD = 'HEAD'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = false
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
# See Net::HTTP for usage examples.
|
|
class Net::HTTP::Post < Net::HTTPRequest
|
|
METHOD = 'POST'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
# See Net::HTTP for usage examples.
|
|
class Net::HTTP::Put < Net::HTTPRequest
|
|
METHOD = 'PUT'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
# See Net::HTTP for usage examples.
|
|
class Net::HTTP::Delete < Net::HTTPRequest
|
|
METHOD = 'DELETE'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Options < Net::HTTPRequest
|
|
METHOD = 'OPTIONS'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = false
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Trace < Net::HTTPRequest
|
|
METHOD = 'TRACE'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
#
|
|
# PATCH method --- RFC5789
|
|
#
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Patch < Net::HTTPRequest
|
|
METHOD = 'PATCH'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
#
|
|
# WebDAV methods --- RFC2518
|
|
#
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Propfind < Net::HTTPRequest
|
|
METHOD = 'PROPFIND'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Proppatch < Net::HTTPRequest
|
|
METHOD = 'PROPPATCH'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Mkcol < Net::HTTPRequest
|
|
METHOD = 'MKCOL'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Copy < Net::HTTPRequest
|
|
METHOD = 'COPY'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Move < Net::HTTPRequest
|
|
METHOD = 'MOVE'
|
|
REQUEST_HAS_BODY = false
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Lock < Net::HTTPRequest
|
|
METHOD = 'LOCK'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|
|
# See Net::HTTPGenericRequest for attributes and methods.
|
|
class Net::HTTP::Unlock < Net::HTTPRequest
|
|
METHOD = 'UNLOCK'
|
|
REQUEST_HAS_BODY = true
|
|
RESPONSE_HAS_BODY = true
|
|
end
|
|
|