mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
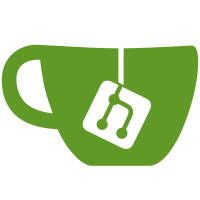
typo. * test/-ext-/old_thread_select/test_old_thread_select.rb (TestOldThreadSelect#test_old_select_signal_safe): use SIGINT instead of SIGUSR1 because the former is general and the latter is platform dependent. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@33130 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
69 lines
1.6 KiB
Ruby
69 lines
1.6 KiB
Ruby
require 'test/unit'
|
|
|
|
class TestOldThreadSelect < Test::Unit::TestCase
|
|
require '-test-/old_thread_select/old_thread_select'
|
|
|
|
def with_pipe
|
|
r, w = IO.pipe
|
|
begin
|
|
yield r, w
|
|
ensure
|
|
r.close unless r.closed?
|
|
w.close unless w.closed?
|
|
end
|
|
end
|
|
|
|
def test_old_select_read_timeout
|
|
with_pipe do |r, w|
|
|
t0 = Time.now
|
|
rc = IO.old_thread_select([r.fileno], nil, nil, 0.001)
|
|
diff = Time.now - t0
|
|
assert_equal 0, rc
|
|
assert diff > 0.001, "returned too early"
|
|
end
|
|
end
|
|
|
|
def test_old_select_read_write_check
|
|
with_pipe do |r, w|
|
|
w.syswrite('.')
|
|
rc = IO.old_thread_select([r.fileno], nil, nil, nil)
|
|
assert_equal 1, rc
|
|
|
|
rc = IO.old_thread_select([r.fileno], [w.fileno], nil, nil)
|
|
assert_equal 2, rc
|
|
|
|
assert_equal '.', r.read(1)
|
|
|
|
rc = IO.old_thread_select([r.fileno], [w.fileno], nil, nil)
|
|
assert_equal 1, rc
|
|
end
|
|
end
|
|
|
|
def test_old_select_signal_safe
|
|
return unless Process.respond_to?(:kill)
|
|
received = false
|
|
trap(:INT) { received = true }
|
|
main = Thread.current
|
|
thr = Thread.new do
|
|
Thread.pass until main.stop?
|
|
Process.kill(:INT, $$)
|
|
true
|
|
end
|
|
|
|
rc = nil
|
|
t0 = Time.now
|
|
with_pipe do |r,w|
|
|
assert_nothing_raised do
|
|
rc = IO.old_thread_select([r.fileno], nil, nil, 1)
|
|
end
|
|
end
|
|
|
|
diff = Time.now - t0
|
|
assert diff >= 1.0, "interrupted or short wait"
|
|
assert_equal 0, rc
|
|
assert_equal true, thr.value
|
|
assert received, "SIGINT not received"
|
|
ensure
|
|
trap(:INT, "DEFAULT")
|
|
end
|
|
end
|