mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
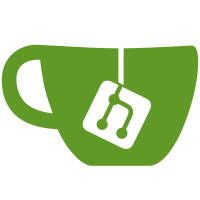
This removes the related tests, and puts the related specs behind version guards. This affects all code in lib, including some libraries that may want to support older versions of Ruby.
53 lines
1.3 KiB
Ruby
53 lines
1.3 KiB
Ruby
# frozen_string_literal: false
|
|
require 'test/unit'
|
|
require '-test-/string'
|
|
require_relative '../symbol/noninterned_name'
|
|
|
|
class Test_String_Fstring < Test::Unit::TestCase
|
|
include Test_Symbol::NonInterned
|
|
|
|
def assert_fstring(str)
|
|
fstr = Bug::String.fstring(str)
|
|
yield str
|
|
yield fstr
|
|
end
|
|
|
|
def test_instance_variable
|
|
str = __method__.to_s * 3
|
|
str.instance_variable_set(:@test, 42)
|
|
str.freeze
|
|
assert_fstring(str) {|s| assert_send([s, :instance_variable_defined?, :@test])}
|
|
end
|
|
|
|
def test_singleton_method
|
|
str = __method__.to_s * 3
|
|
def str.foo
|
|
end
|
|
str.freeze
|
|
assert_fstring(str) {|s| assert_send([s, :respond_to?, :foo])}
|
|
end
|
|
|
|
def test_singleton_class
|
|
str = noninterned_name
|
|
fstr = Bug::String.fstring(str)
|
|
assert_raise(TypeError) {fstr.singleton_class}
|
|
end
|
|
|
|
class S < String
|
|
end
|
|
|
|
def test_subclass
|
|
str = S.new(__method__.to_s * 3)
|
|
str.freeze
|
|
assert_fstring(str) {|s| assert_instance_of(S, s)}
|
|
end
|
|
|
|
def test_shared_string_safety
|
|
_unused = -('a' * 30).force_encoding(Encoding::ASCII)
|
|
str = ('a' * 30).force_encoding(Encoding::ASCII).taint
|
|
frozen_str = Bug::String.rb_str_new_frozen(str)
|
|
assert_fstring(frozen_str) {|s| assert_equal(str, s)}
|
|
GC.start
|
|
assert_equal('a' * 30, str, "[Bug #16151]")
|
|
end
|
|
end
|