mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
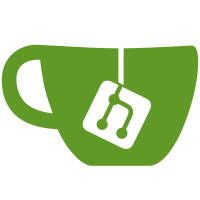
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@14048 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
41 lines
896 B
Ruby
41 lines
896 B
Ruby
require 'test/unit'
|
|
|
|
class TestProcess < Test::Unit::TestCase
|
|
def test_rlimit_availability
|
|
begin
|
|
Process.getrlimit(nil)
|
|
rescue NotImplementedError
|
|
assert_raise(NotImplementedError) { Process.setrlimit }
|
|
rescue TypeError
|
|
assert_raise(ArgumentError) { Process.setrlimit }
|
|
end
|
|
end
|
|
|
|
def rlimit_exist?
|
|
Process.getrlimit(nil)
|
|
rescue NotImplementedError
|
|
return false
|
|
rescue TypeError
|
|
return true
|
|
end
|
|
|
|
def test_rlimit_nofile
|
|
return unless rlimit_exist?
|
|
pid = fork {
|
|
cur_nofile, max_nofile = Process.getrlimit(Process::RLIMIT_NOFILE)
|
|
begin
|
|
Process.setrlimit(Process::RLIMIT_NOFILE, 0, max_nofile)
|
|
rescue Errno::EINVAL
|
|
exit 0
|
|
end
|
|
begin
|
|
IO.pipe
|
|
rescue Errno::EMFILE
|
|
exit 0
|
|
end
|
|
exit 1
|
|
}
|
|
Process.wait pid
|
|
assert_equal(0, $?.to_i, "#{$?}")
|
|
end
|
|
end
|