mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
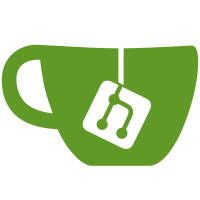
[ruby-core:29104] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@27095 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
124 lines
3.1 KiB
Ruby
124 lines
3.1 KiB
Ruby
require_relative '../helper'
|
|
|
|
module Psych
|
|
module Visitors
|
|
class TestEmitter < TestCase
|
|
def setup
|
|
super
|
|
@io = StringIO.new
|
|
@visitor = Visitors::Emitter.new @io
|
|
end
|
|
|
|
def test_stream
|
|
s = Nodes::Stream.new
|
|
@visitor.accept s
|
|
assert_equal '', @io.string
|
|
end
|
|
|
|
def test_document
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new [1,1]
|
|
scalar = Nodes::Scalar.new 'hello world'
|
|
|
|
doc.children << scalar
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/1.1/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
|
|
def test_document_implicit_end
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
mapping = Nodes::Mapping.new
|
|
mapping.children << Nodes::Scalar.new('key')
|
|
mapping.children << Nodes::Scalar.new('value')
|
|
doc.children << mapping
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/key: value/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
assert(/\.\.\./ !~ s.to_yaml)
|
|
end
|
|
|
|
def test_scalar
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
scalar = Nodes::Scalar.new 'hello world'
|
|
|
|
doc.children << scalar
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/hello/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
|
|
def test_scalar_with_tag
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
scalar = Nodes::Scalar.new 'hello world', nil, '!str', false, false, 5
|
|
|
|
doc.children << scalar
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/str/, @io.string)
|
|
assert_match(/hello/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
|
|
def test_sequence
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
scalar = Nodes::Scalar.new 'hello world'
|
|
seq = Nodes::Sequence.new
|
|
|
|
seq.children << scalar
|
|
doc.children << seq
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/- hello/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
|
|
def test_mapping
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
mapping = Nodes::Mapping.new
|
|
mapping.children << Nodes::Scalar.new('key')
|
|
mapping.children << Nodes::Scalar.new('value')
|
|
doc.children << mapping
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/key: value/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
|
|
def test_alias
|
|
s = Nodes::Stream.new
|
|
doc = Nodes::Document.new
|
|
mapping = Nodes::Mapping.new
|
|
mapping.children << Nodes::Scalar.new('key', 'A')
|
|
mapping.children << Nodes::Alias.new('A')
|
|
doc.children << mapping
|
|
s.children << doc
|
|
|
|
@visitor.accept s
|
|
|
|
assert_match(/&A key: \*A/, @io.string)
|
|
assert_equal @io.string, s.to_yaml
|
|
end
|
|
end
|
|
end
|
|
end
|