mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
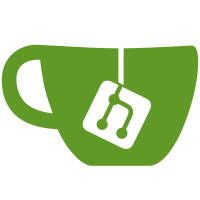
* For easier modifications of ruby/spec by MRI developers. * .gitignore: track changes under spec. * spec/mspec, spec/rubyspec: add in-tree mspec and ruby/spec. These files can therefore be updated like any other file in MRI. Instructions are provided in spec/README. [Feature #13156] [ruby-core:79246] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@58595 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
68 lines
1.7 KiB
Ruby
68 lines
1.7 KiB
Ruby
require File.expand_path('../../../spec_helper', __FILE__)
|
|
require File.expand_path('../fixtures/classes', __FILE__)
|
|
require 'matrix'
|
|
|
|
describe "Matrix#empty?" do
|
|
it "returns true when the Matrix is empty" do
|
|
Matrix[ ].empty?.should be_true
|
|
Matrix[ [], [], [] ].empty?.should be_true
|
|
Matrix[ [], [], [] ].transpose.empty?.should be_true
|
|
end
|
|
|
|
it "returns false when the Matrix has elements" do
|
|
Matrix[ [1, 2] ].empty?.should be_false
|
|
Matrix[ [1], [2] ].empty?.should be_false
|
|
end
|
|
|
|
it "doesn't accept any parameter" do
|
|
lambda{
|
|
Matrix[ [1, 2] ].empty?(42)
|
|
}.should raise_error(ArgumentError)
|
|
end
|
|
end
|
|
|
|
describe "Matrix.empty" do
|
|
it "returns an empty matrix of the requested size" do
|
|
m = Matrix.empty(3, 0)
|
|
m.row_size.should == 3
|
|
m.column_size.should == 0
|
|
|
|
m = Matrix.empty(0, 3)
|
|
m.row_size.should == 0
|
|
m.column_size.should == 3
|
|
end
|
|
|
|
it "has arguments defaulting to 0" do
|
|
Matrix.empty.should == Matrix.empty(0, 0)
|
|
Matrix.empty(42).should == Matrix.empty(42, 0)
|
|
end
|
|
|
|
it "does not accept more than two parameters" do
|
|
lambda{
|
|
Matrix.empty(1, 2, 3)
|
|
}.should raise_error(ArgumentError)
|
|
end
|
|
|
|
it "raises an error if both dimensions are > 0" do
|
|
lambda{
|
|
Matrix.empty(1, 2)
|
|
}.should raise_error(ArgumentError)
|
|
end
|
|
|
|
it "raises an error if any dimension is < 0" do
|
|
lambda{
|
|
Matrix.empty(-2, 0)
|
|
}.should raise_error(ArgumentError)
|
|
|
|
lambda{
|
|
Matrix.empty(0, -2)
|
|
}.should raise_error(ArgumentError)
|
|
end
|
|
|
|
end
|
|
|
|
describe "for a subclass of Matrix" do
|
|
it "returns an instance of that subclass" do
|
|
MatrixSub.empty(0, 1).should be_an_instance_of(MatrixSub)
|
|
end
|
|
end
|