mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
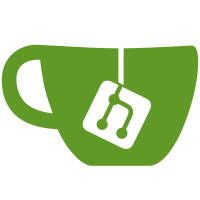
* Enable Style/MethodDefParentheses in Rubocop https://github.com/rubygems/rubygems/pull/2478 * Enable Style/MultilineIfThen in Rubocop https://github.com/rubygems/rubygems/pull/2479 * Fix required_ruby_version with prereleases and improve error message https://github.com/rubygems/rubygems/pull/2344 * Fix bundler rubygems binstub not properly looking for bundler https://github.com/rubygems/rubygems/pull/2426 git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@65904 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
64 lines
1.3 KiB
Ruby
64 lines
1.3 KiB
Ruby
# frozen_string_literal: true
|
|
##
|
|
# IO wrapper that creates digests of contents written to the IO it wraps.
|
|
|
|
class Gem::Package::DigestIO
|
|
|
|
##
|
|
# Collected digests for wrapped writes.
|
|
#
|
|
# {
|
|
# 'SHA1' => #<OpenSSL::Digest: [...]>,
|
|
# 'SHA512' => #<OpenSSL::Digest: [...]>,
|
|
# }
|
|
|
|
attr_reader :digests
|
|
|
|
##
|
|
# Wraps +io+ and updates digest for each of the digest algorithms in
|
|
# the +digests+ Hash. Returns the digests hash. Example:
|
|
#
|
|
# io = StringIO.new
|
|
# digests = {
|
|
# 'SHA1' => OpenSSL::Digest.new('SHA1'),
|
|
# 'SHA512' => OpenSSL::Digest.new('SHA512'),
|
|
# }
|
|
#
|
|
# Gem::Package::DigestIO.wrap io, digests do |digest_io|
|
|
# digest_io.write "hello"
|
|
# end
|
|
#
|
|
# digests['SHA1'].hexdigest #=> "aaf4c61d[...]"
|
|
# digests['SHA512'].hexdigest #=> "9b71d224[...]"
|
|
|
|
def self.wrap(io, digests)
|
|
digest_io = new io, digests
|
|
|
|
yield digest_io
|
|
|
|
return digests
|
|
end
|
|
|
|
##
|
|
# Creates a new DigestIO instance. Using ::wrap is recommended, see the
|
|
# ::wrap documentation for documentation of +io+ and +digests+.
|
|
|
|
def initialize(io, digests)
|
|
@io = io
|
|
@digests = digests
|
|
end
|
|
|
|
##
|
|
# Writes +data+ to the underlying IO and updates the digests
|
|
|
|
def write(data)
|
|
result = @io.write data
|
|
|
|
@digests.each do |_, digest|
|
|
digest << data
|
|
end
|
|
|
|
result
|
|
end
|
|
|
|
end
|