mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
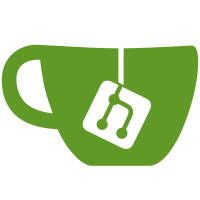
* lib/soap/mapping/wsdl*.rb * lib/wsdl/soap/element.rb * lib/wsdl/xmlSchema/simpleContent.rb * modified files: * lib/soap/* * lib/wsdl/* * lib/xsd/* * test/soap/* * test/wsdl/* * test/xsd/* * summary * imported from the soap4r repository. Version: 1.5.3-ruby1.8.2 * added several XSD basetype support: nonPositiveInteger, negativeInteger, nonNegativeInteger, unsignedLong, unsignedInt, unsignedShort, unsignedByte, positiveInteger * HTTP client connection/send/receive timeout support. * HTTP client/server gzipped content encoding support. * improved WSDL schema definition support; still is far from complete, but is making step by step improovement. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@7612 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
84 lines
1.5 KiB
Ruby
84 lines
1.5 KiB
Ruby
# WSDL4R - WSDL port definition.
|
|
# Copyright (C) 2002, 2003 NAKAMURA, Hiroshi <nahi@ruby-lang.org>.
|
|
|
|
# This program is copyrighted free software by NAKAMURA, Hiroshi. You can
|
|
# redistribute it and/or modify it under the same terms of Ruby's license;
|
|
# either the dual license version in 2003, or any later version.
|
|
|
|
|
|
require 'wsdl/info'
|
|
|
|
|
|
module WSDL
|
|
|
|
|
|
class Port < Info
|
|
attr_reader :name # required
|
|
attr_reader :binding # required
|
|
attr_reader :soap_address
|
|
|
|
def initialize
|
|
super
|
|
@name = nil
|
|
@binding = nil
|
|
@soap_address = nil
|
|
end
|
|
|
|
def targetnamespace
|
|
parent.targetnamespace
|
|
end
|
|
|
|
def porttype
|
|
root.porttype(find_binding.type)
|
|
end
|
|
|
|
def find_binding
|
|
root.binding(@binding)
|
|
end
|
|
|
|
def inputoperation_map
|
|
result = {}
|
|
find_binding.operations.each do |op_bind|
|
|
op_info = op_bind.soapoperation.input_info
|
|
result[op_info.op_name] = op_info
|
|
end
|
|
result
|
|
end
|
|
|
|
def outputoperation_map
|
|
result = {}
|
|
find_binding.operations.each do |op_bind|
|
|
op_info = op_bind.soapoperation.output_info
|
|
result[op_info.op_name] = op_info
|
|
end
|
|
result
|
|
end
|
|
|
|
def parse_element(element)
|
|
case element
|
|
when SOAPAddressName
|
|
o = WSDL::SOAP::Address.new
|
|
@soap_address = o
|
|
o
|
|
when DocumentationName
|
|
o = Documentation.new
|
|
o
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
|
|
def parse_attr(attr, value)
|
|
case attr
|
|
when NameAttrName
|
|
@name = XSD::QName.new(targetnamespace, value.source)
|
|
when BindingAttrName
|
|
@binding = value
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
end
|
|
|
|
|
|
end
|