mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
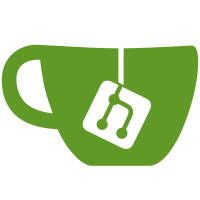
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@518 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
50 lines
893 B
Ruby
50 lines
893 B
Ruby
#!/usr/local/bin/ruby
|
|
# This script generates a counter with start and stop buttons.
|
|
|
|
require "tk"
|
|
$label = TkLabel.new {
|
|
text '0.00'
|
|
relief 'raised'
|
|
width 10
|
|
pack('side'=>'bottom', 'fill'=>'both')
|
|
}
|
|
|
|
TkButton.new {
|
|
text 'Start'
|
|
command proc {
|
|
if $stopped
|
|
$stopped = FALSE
|
|
tick
|
|
end
|
|
}
|
|
pack('side'=>'left','fill'=>'both','expand'=>'yes')
|
|
}
|
|
TkButton.new {
|
|
text 'Stop'
|
|
command proc{
|
|
exit if $stopped
|
|
$stopped = TRUE
|
|
}
|
|
pack('side'=>'right','fill'=>'both','expand'=>'yes')
|
|
}
|
|
|
|
$seconds=0
|
|
$hundredths=0
|
|
$stopped=TRUE
|
|
|
|
def tick
|
|
if $stopped then return end
|
|
Tk.after 50, proc{tick}
|
|
$hundredths+=5
|
|
if $hundredths >= 100
|
|
$hundredths=0
|
|
$seconds+=1
|
|
end
|
|
$label.text format("%d.%02d", $seconds, $hundredths)
|
|
end
|
|
|
|
root = Tk.root
|
|
root.bind "Control-c", proc{root.destroy}
|
|
root.bind "Control-q", proc{root.destroy}
|
|
Tk.root.focus
|
|
Tk.mainloop
|