mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
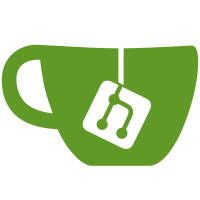
/var/folders/b0/9hgj_tyx10zgkcbyx3_j2dlr0000gn/T//_ruby_mjit_p72619u0.c:37:58: warning: incompatible integer to pointer conversion passing 'long' to parameter of type 'const struct rb_call_info *' [-Wint-conversion] vm_caller_setup_arg_block(ec, reg_cfp, &calling, 0x7ff6b2e10ca0, 0x7ff6b3847108, FALSE); ^~~~~~~~~~~~~~ /Users/kokubun/.rbenv/versions/ruby-svn/include/ruby-2.6.0/x86_64-darwin16/rb_mjit_min_header-2.6.0.h:15613:66: note: passing argument to parameter 'ci' here struct rb_calling_info *calling, const struct rb_call_info *ci, rb_iseq_t *blockiseq, const int is_super) ^ /var/folders/b0/9hgj_tyx10zgkcbyx3_j2dlr0000gn/T//_ruby_mjit_p72619u0.c:37:74: warning: incompatible integer to pointer conversion passing 'long' to parameter of type 'rb_iseq_t *' (aka 'struct rb_iseq_struct *') [-Wint-conversion] vm_caller_setup_arg_block(ec, reg_cfp, &calling, 0x7ff6b2e10ca0, 0x7ff6b3847108, FALSE); ^~~~~~~~~~~~~~ /Users/kokubun/.rbenv/versions/ruby-svn/include/ruby-2.6.0/x86_64-darwin16/rb_mjit_min_header-2.6.0.h:15613:81: note: passing argument to parameter 'blockiseq' here struct rb_calling_info *calling, const struct rb_call_info *ci, rb_iseq_t *blockiseq, const int is_super) ^ 2 warnings generated. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@63298 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
92 lines
4.9 KiB
Text
92 lines
4.9 KiB
Text
% # -*- mode:c; style:ruby; coding: utf-8; indent-tabs-mode: nil -*-
|
|
% # Copyright (c) 2018 Takashi Kokubun. All rights reserved.
|
|
% #
|
|
% # This file is a part of the programming language Ruby. Permission is hereby
|
|
% # granted, to either redistribute and/or modify this file, provided that the
|
|
% # conditions mentioned in the file COPYING are met. Consult the file for
|
|
% # details.
|
|
%
|
|
% # Optimized case of send / opt_send_without_block instructions.
|
|
{
|
|
% # compiler: Prepare operands which may be used by `insn.call_attribute`
|
|
% insn.opes.each_with_index do |ope, i|
|
|
MAYBE_UNUSED(<%= ope.fetch(:decl) %>) = (<%= ope.fetch(:type) %>)operands[<%= i %>];
|
|
% end
|
|
%
|
|
if (has_valid_method_type(cc)) {
|
|
const rb_iseq_t *iseq;
|
|
unsigned int argc = ci->orig_argc; /* unlike `ci->orig_argc`, `argc` may include blockarg */
|
|
% if insn.name == 'send'
|
|
argc += ((ci->flag & VM_CALL_ARGS_BLOCKARG) ? 1 : 0);
|
|
% end
|
|
|
|
if (cc->me->def->type == VM_METHOD_TYPE_ISEQ && inlinable_iseq_p(ci, cc, iseq = rb_iseq_check(cc->me->def->body.iseq.iseqptr))) {
|
|
int param_size = iseq->body->param.size; /* TODO: check calling->argc for argument_arity_error */
|
|
|
|
fprintf(f, "{\n");
|
|
% # JIT: Declare stack_size to be used in some macro of _mjit_compile_insn_body.erb
|
|
if (status->local_stack_p) {
|
|
fprintf(f, " MAYBE_UNUSED(unsigned int) stack_size = %u;\n", b->stack_size);
|
|
}
|
|
|
|
% # JIT: move sp and pc if necessary
|
|
<%= render 'mjit_compile_pc_and_sp', locals: { insn: insn } -%>
|
|
|
|
% # JIT: Invalidate call cache if it requires vm_search_method. This allows to inline some of following things.
|
|
fprintf(f, " if (UNLIKELY(GET_GLOBAL_METHOD_STATE() != %"PRI_SERIALT_PREFIX"u ||\n", cc->method_state);
|
|
fprintf(f, " RCLASS_SERIAL(CLASS_OF(stack[%d])) != %"PRI_SERIALT_PREFIX"u)) {\n", b->stack_size - 1 - argc, cc->class_serial);
|
|
fprintf(f, " reg_cfp->pc = original_body_iseq + %d;\n", pos);
|
|
fprintf(f, " goto cancel;\n");
|
|
fprintf(f, " }\n");
|
|
|
|
% # JIT: Print insn body in insns.def
|
|
fprintf(f, " {\n");
|
|
fprintf(f, " struct rb_calling_info calling;\n");
|
|
% if insn.name == 'send'
|
|
fprintf(f, " vm_caller_setup_arg_block(ec, reg_cfp, &calling, (CALL_INFO)0x%"PRIxVALUE", (rb_iseq_t *)0x%"PRIxVALUE", FALSE);\n", operands[0], operands[2]);
|
|
% else
|
|
fprintf(f, " calling.block_handler = VM_BLOCK_HANDLER_NONE;\n");
|
|
% end
|
|
fprintf(f, " calling.argc = %d;\n", ci->orig_argc);
|
|
fprintf(f, " calling.recv = stack[%d];\n", b->stack_size - 1 - argc);
|
|
|
|
% # JIT: Special CALL_METHOD. Inline vm_call_iseq_setup_normal for vm_call_iseq_setup_func FASTPATH. TODO: modify VM to share code with here
|
|
fprintf(f, " {\n");
|
|
fprintf(f, " VALUE v;\n");
|
|
fprintf(f, " VALUE *argv = reg_cfp->sp - calling.argc;\n");
|
|
fprintf(f, " reg_cfp->sp = argv - 1;\n"); /* recv */
|
|
fprintf(f, " vm_push_frame(ec, (const rb_iseq_t *)0x%"PRIxVALUE", VM_FRAME_MAGIC_METHOD | VM_ENV_FLAG_LOCAL, calling.recv, "
|
|
"calling.block_handler, 0x%"PRIxVALUE", (const VALUE *)0x%"PRIxVALUE", argv + %d, %d, %d);\n",
|
|
(VALUE)iseq, (VALUE)cc->me, (VALUE)iseq->body->iseq_encoded, param_size, iseq->body->local_table_size - param_size, iseq->body->stack_max);
|
|
if (iseq->body->catch_except_p) {
|
|
fprintf(f, " VM_ENV_FLAGS_SET(ec->cfp->ep, VM_FRAME_FLAG_FINISH);\n");
|
|
fprintf(f, " v = vm_exec(ec, TRUE);\n");
|
|
}
|
|
else {
|
|
fprintf(f, " if ((v = mjit_exec(ec)) == Qundef) {\n");
|
|
fprintf(f, " VM_ENV_FLAGS_SET(ec->cfp->ep, VM_FRAME_FLAG_FINISH);\n"); /* This is vm_call0_body's code after vm_call_iseq_setup */
|
|
fprintf(f, " v = vm_exec(ec, FALSE);\n");
|
|
fprintf(f, " }\n");
|
|
}
|
|
fprintf(f, " stack[%d] = v;\n", b->stack_size - argc - 1);
|
|
fprintf(f, " }\n");
|
|
|
|
fprintf(f, " }\n");
|
|
|
|
% # JIT: We should evaluate ISeq modified for TracePoint if it's enabled. Note: This is slow.
|
|
fprintf(f, " if (UNLIKELY(ruby_vm_event_enabled_flags & ISEQ_TRACE_EVENTS)) {\n");
|
|
fprintf(f, " reg_cfp->sp = (VALUE *)reg_cfp->bp + %d;\n", b->stack_size + (int)<%= insn.call_attribute('sp_inc') %> + 1);
|
|
if (!body->catch_except_p) {
|
|
fprintf(f, " reg_cfp->pc = original_body_iseq + %d;\n", next_pos);
|
|
}
|
|
fprintf(f, " goto cancel;\n");
|
|
fprintf(f, " }\n");
|
|
|
|
% # compiler: Move JIT compiler's internal stack pointer
|
|
b->stack_size += <%= insn.call_attribute('sp_inc') %>;
|
|
|
|
fprintf(f, "}\n");
|
|
break;
|
|
}
|
|
}
|
|
}
|