mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
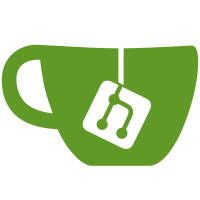
* ext/fiddle/closure.c: use directly declaration for standalone gem without internal.h. * Specify frozen string literal is true. * Update gemspec configuration for release version. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@59854 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
49 lines
1.2 KiB
Ruby
49 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
module Fiddle
|
|
class Closure
|
|
|
|
# the C type of the return of the FFI closure
|
|
attr_reader :ctype
|
|
|
|
# arguments of the FFI closure
|
|
attr_reader :args
|
|
|
|
# Extends Fiddle::Closure to allow for building the closure in a block
|
|
class BlockCaller < Fiddle::Closure
|
|
|
|
# == Description
|
|
#
|
|
# Construct a new BlockCaller object.
|
|
#
|
|
# * +ctype+ is the C type to be returned
|
|
# * +args+ are passed the callback
|
|
# * +abi+ is the abi of the closure
|
|
#
|
|
# If there is an error in preparing the +ffi_cif+ or +ffi_prep_closure+,
|
|
# then a RuntimeError will be raised.
|
|
#
|
|
# == Example
|
|
#
|
|
# include Fiddle
|
|
#
|
|
# cb = Closure::BlockCaller.new(TYPE_INT, [TYPE_INT]) do |one|
|
|
# one
|
|
# end
|
|
#
|
|
# func = Function.new(cb, [TYPE_INT], TYPE_INT)
|
|
#
|
|
def initialize ctype, args, abi = Fiddle::Function::DEFAULT, &block
|
|
super(ctype, args, abi)
|
|
@block = block
|
|
end
|
|
|
|
# Calls the constructed BlockCaller, with +args+
|
|
#
|
|
# For an example see Fiddle::Closure::BlockCaller.new
|
|
#
|
|
def call *args
|
|
@block.call(*args)
|
|
end
|
|
end
|
|
end
|
|
end
|