mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
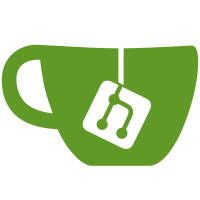
Now that we can say for sure if an instruction calls a method or not internally, it is now possible to reroute the bugs that forced us to revert the "move PC around" optimization. First try: r62051 Reverted: r63763 See also: r63999 ---- trunk: ruby 2.6.0dev (2018-09-13 trunk 64736) [x86_64-darwin15] ours: ruby 2.6.0dev (2018-09-13 trunk 64736) [x86_64-darwin15] last_commit=move ADD_PC around (take 2) Calculating ------------------------------------- trunk ours so_ackermann 1.884 2.278 i/s - 1.000 times in 0.530926s 0.438935s so_array 1.178 1.157 i/s - 1.000 times in 0.848786s 0.864467s so_binary_trees 0.176 0.177 i/s - 1.000 times in 5.683895s 5.657707s so_concatenate 0.220 0.221 i/s - 1.000 times in 4.546896s 4.518949s so_count_words 6.729 6.470 i/s - 1.000 times in 0.148602s 0.154561s so_exception 3.324 3.688 i/s - 1.000 times in 0.300872s 0.271147s so_fannkuch 0.546 0.968 i/s - 1.000 times in 1.831328s 1.033376s so_fasta 0.541 0.547 i/s - 1.000 times in 1.849923s 1.827091s so_k_nucleotide 0.800 0.777 i/s - 1.000 times in 1.250635s 1.286295s so_lists 2.101 1.848 i/s - 1.000 times in 0.475954s 0.541095s so_mandelbrot 0.435 0.408 i/s - 1.000 times in 2.299328s 2.450535s so_matrix 1.946 1.912 i/s - 1.000 times in 0.513872s 0.523076s so_meteor_contest 0.311 0.317 i/s - 1.000 times in 3.219297s 3.152052s so_nbody 0.746 0.703 i/s - 1.000 times in 1.339815s 1.423441s so_nested_loop 0.899 0.901 i/s - 1.000 times in 1.111767s 1.109555s so_nsieve 0.559 0.579 i/s - 1.000 times in 1.787763s 1.726552s so_nsieve_bits 0.435 0.428 i/s - 1.000 times in 2.296282s 2.333852s so_object 1.368 1.442 i/s - 1.000 times in 0.731237s 0.693684s so_partial_sums 0.616 0.546 i/s - 1.000 times in 1.623592s 1.833097s so_pidigits 0.831 0.832 i/s - 1.000 times in 1.203117s 1.202334s so_random 2.934 2.724 i/s - 1.000 times in 0.340791s 0.367150s so_reverse_complement 0.583 0.866 i/s - 1.000 times in 1.714144s 1.154615s so_sieve 1.829 2.081 i/s - 1.000 times in 0.546607s 0.480562s so_spectralnorm 0.524 0.558 i/s - 1.000 times in 1.908716s 1.792382s git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@64737 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
105 lines
4.5 KiB
Text
105 lines
4.5 KiB
Text
% # -*- mode:c; style:ruby; coding: utf-8; indent-tabs-mode: nil -*-
|
|
% # Copyright (c) 2018 Takashi Kokubun. All rights reserved.
|
|
% #
|
|
% # This file is a part of the programming language Ruby. Permission is hereby
|
|
% # granted, to either redistribute and/or modify this file, provided that the
|
|
% # conditions mentioned in the file COPYING are met. Consult the file for
|
|
% # details.
|
|
%
|
|
% to_cstr = lambda do |line|
|
|
% normalized = line.gsub(/\t/, ' ' * 8)
|
|
% indented = normalized.sub(/\A(?!#)/, ' ') # avoid indenting preprocessor
|
|
% rstring2cstr(indented.rstrip).sub(/"\z/, '\\n"')
|
|
% end
|
|
%
|
|
% #
|
|
% # Expand simple macro, which doesn't require dynamic C code.
|
|
% #
|
|
% expand_simple_macros = lambda do |arg_expr|
|
|
% arg_expr.dup.tap do |expr|
|
|
% # For `leave`. We can't proceed next ISeq in the same JIT function.
|
|
% expr.gsub!(/^(?<indent>\s*)RESTORE_REGS\(\);\n/) do
|
|
% indent = Regexp.last_match[:indent]
|
|
% <<-RESTORE_REGS.gsub(/^ +/, '')
|
|
% #if OPT_CALL_THREADED_CODE
|
|
% #{indent}rb_ec_thread_ptr(ec)->retval = val;
|
|
% #{indent}return 0;
|
|
% #else
|
|
% #{indent}return val;
|
|
% #endif
|
|
% RESTORE_REGS
|
|
% end
|
|
% end
|
|
% end
|
|
%
|
|
% #
|
|
% # Print a body of insn, but with macro expansion.
|
|
% #
|
|
% expand_simple_macros.call(insn.expr.expr).each_line do |line|
|
|
% #
|
|
% # Expand dynamic macro here (only JUMP for now)
|
|
% #
|
|
% # TODO: support combination of following macros in the same line
|
|
% case line
|
|
% when /\A\s+JUMP\((?<dest>[^)]+)\);\s+\z/
|
|
% dest = Regexp.last_match[:dest]
|
|
%
|
|
% if insn.name == 'opt_case_dispatch' # special case... TODO: use another macro to avoid checking name
|
|
{
|
|
struct case_dispatch_var arg;
|
|
arg.f = f;
|
|
arg.base_pos = pos + insn_len(insn);
|
|
arg.last_value = Qundef;
|
|
|
|
fprintf(f, " switch (<%= dest %>) {\n");
|
|
st_foreach(RHASH_TBL_RAW(hash), compile_case_dispatch_each, (VALUE)&arg);
|
|
fprintf(f, " case %lu:\n", else_offset);
|
|
fprintf(f, " goto label_%lu;\n", arg.base_pos + else_offset);
|
|
fprintf(f, " }\n");
|
|
}
|
|
% else
|
|
% # Before we `goto` next insn, we need to set return values, especially for getinlinecache
|
|
% insn.rets.reverse_each.with_index do |ret, i|
|
|
% # TOPN(n) = ...
|
|
fprintf(f, " stack[%d] = <%= ret.fetch(:name) %>;\n", b->stack_size + (int)<%= insn.call_attribute('sp_inc') %> - <%= i + 1 %>);
|
|
% end
|
|
%
|
|
next_pos = pos + insn_len(insn) + (unsigned int)<%= dest %>;
|
|
fprintf(f, " goto label_%d;\n", next_pos);
|
|
% end
|
|
% when /\A\s+CALL_SIMPLE_METHOD\(\);\s+\z/
|
|
% # For `opt_xxx`'s fallbacks.
|
|
if (status->local_stack_p) {
|
|
fprintf(f, " reg_cfp->sp = (VALUE *)reg_cfp->bp + %d;\n", b->stack_size + 1);
|
|
}
|
|
fprintf(f, " reg_cfp->pc = original_body_iseq + %d;\n", pos);
|
|
fprintf(f, " goto cancel;\n");
|
|
% else
|
|
% if insn.handles_sp?
|
|
% # If insn.handles_sp? is true, cfp->sp might be changed inside insns (like vm_caller_setup_arg_block)
|
|
% # and thus we need to use cfp->sp, even when local_stack_p is TRUE. When insn.handles_sp? is true,
|
|
% # cfp->sp should be available too because _mjit_compile_pc_and_sp.erb sets it.
|
|
fprintf(f, <%= to_cstr.call(line) %>);
|
|
% else
|
|
% # If local_stack_p is TRUE and insn.handles_sp? is false, stack values are only available in local variables
|
|
% # for stack. So we need to replace those macros if local_stack_p is TRUE here.
|
|
% case line
|
|
% when /\bGET_SP\(\)/
|
|
% # reg_cfp->sp
|
|
fprintf(f, <%= to_cstr.call(line.sub(/\bGET_SP\(\)/, '%s')) %>, (status->local_stack_p ? "(stack + stack_size)" : "GET_SP()"));
|
|
% when /\bSTACK_ADDR_FROM_TOP\((?<num>[^)]+)\)/
|
|
% # #define STACK_ADDR_FROM_TOP(n) (GET_SP()-(n))
|
|
% num = Regexp.last_match[:num]
|
|
fprintf(f, <%= to_cstr.call(line.sub(/\bSTACK_ADDR_FROM_TOP\(([^)]+)\)/, '%s')) %>,
|
|
(status->local_stack_p ? "stack + (stack_size - (<%= num %>))" : "STACK_ADDR_FROM_TOP(<%= num %>)"));
|
|
% when /\bTOPN\((?<num>[^)]+)\)/
|
|
% # #define TOPN(n) (*(GET_SP()-(n)-1))
|
|
% num = Regexp.last_match[:num]
|
|
fprintf(f, <%= to_cstr.call(line.sub(/\bTOPN\(([^)]+)\)/, '%s')) %>,
|
|
(status->local_stack_p ? "*(stack + (stack_size - (<%= num %>) - 1))" : "TOPN(<%= num %>)"));
|
|
% else
|
|
fprintf(f, <%= to_cstr.call(line) %>);
|
|
% end
|
|
% end
|
|
% end
|
|
% end
|