mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
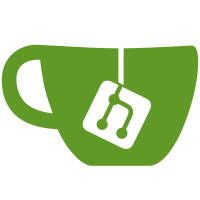
* bin/*, lib/bundler/*, lib/bundler.rb, spec/bundler, man/*: Merge from latest stable branch of bundler/bundler repository and added workaround patches. I will backport them into upstream. * common.mk, defs/gmake.mk: Added `test-bundler` task for test suite of bundler. * tool/sync_default_gems.rb: Added sync task for bundler. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@65509 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
107 lines
2.7 KiB
Ruby
107 lines
2.7 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
RSpec.describe "bundle install with ENV conditionals" do
|
|
describe "when just setting an ENV key as a string" do
|
|
before :each do
|
|
gemfile <<-G
|
|
source "file://#{gem_repo1}"
|
|
|
|
env "BUNDLER_TEST" do
|
|
gem "rack"
|
|
end
|
|
G
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is not set" do
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "includes the gems when the ENV variable is set" do
|
|
ENV["BUNDLER_TEST"] = "1"
|
|
bundle :install
|
|
expect(the_bundle).to include_gems "rack 1.0"
|
|
end
|
|
end
|
|
|
|
describe "when just setting an ENV key as a symbol" do
|
|
before :each do
|
|
gemfile <<-G
|
|
source "file://#{gem_repo1}"
|
|
|
|
env :BUNDLER_TEST do
|
|
gem "rack"
|
|
end
|
|
G
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is not set" do
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "includes the gems when the ENV variable is set" do
|
|
ENV["BUNDLER_TEST"] = "1"
|
|
bundle :install
|
|
expect(the_bundle).to include_gems "rack 1.0"
|
|
end
|
|
end
|
|
|
|
describe "when setting a string to match the env" do
|
|
before :each do
|
|
gemfile <<-G
|
|
source "file://#{gem_repo1}"
|
|
|
|
env "BUNDLER_TEST" => "foo" do
|
|
gem "rack"
|
|
end
|
|
G
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is not set" do
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is set but does not match the condition" do
|
|
ENV["BUNDLER_TEST"] = "1"
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "includes the gems when the ENV variable is set and matches the condition" do
|
|
ENV["BUNDLER_TEST"] = "foo"
|
|
bundle :install
|
|
expect(the_bundle).to include_gems "rack 1.0"
|
|
end
|
|
end
|
|
|
|
describe "when setting a regex to match the env" do
|
|
before :each do
|
|
gemfile <<-G
|
|
source "file://#{gem_repo1}"
|
|
|
|
env "BUNDLER_TEST" => /foo/ do
|
|
gem "rack"
|
|
end
|
|
G
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is not set" do
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "excludes the gems when the ENV variable is set but does not match the condition" do
|
|
ENV["BUNDLER_TEST"] = "fo"
|
|
bundle :install
|
|
expect(the_bundle).not_to include_gems "rack"
|
|
end
|
|
|
|
it "includes the gems when the ENV variable is set and matches the condition" do
|
|
ENV["BUNDLER_TEST"] = "foobar"
|
|
bundle :install
|
|
expect(the_bundle).to include_gems "rack 1.0"
|
|
end
|
|
end
|
|
end
|