mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
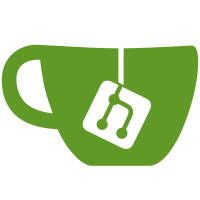
* For easier modifications of ruby/spec by MRI developers. * .gitignore: track changes under spec. * spec/mspec, spec/rubyspec: add in-tree mspec and ruby/spec. These files can therefore be updated like any other file in MRI. Instructions are provided in spec/README. [Feature #13156] [ruby-core:79246] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@58595 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
134 lines
2.3 KiB
Ruby
134 lines
2.3 KiB
Ruby
class NextSpecs
|
|
def self.yielding_method(expected)
|
|
yield.should == expected
|
|
:method_return_value
|
|
end
|
|
|
|
def self.yielding
|
|
yield
|
|
end
|
|
|
|
# The methods below are defined to spec the behavior of the next statement
|
|
# while specifically isolating whether the statement is in an Iter block or
|
|
# not. In a normal spec example, the code is always nested inside a block.
|
|
# Rather than rely on that implicit context in this case, the context is
|
|
# made explicit because of the interaction of next in a loop nested inside
|
|
# an Iter block.
|
|
def self.while_next(arg)
|
|
x = true
|
|
while x
|
|
begin
|
|
ScratchPad << :begin
|
|
x = false
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
|
|
def self.while_within_iter(arg)
|
|
yielding do
|
|
x = true
|
|
while x
|
|
begin
|
|
ScratchPad << :begin
|
|
x = false
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
def self.until_next(arg)
|
|
x = false
|
|
until x
|
|
begin
|
|
ScratchPad << :begin
|
|
x = true
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
|
|
def self.until_within_iter(arg)
|
|
yielding do
|
|
x = false
|
|
until x
|
|
begin
|
|
ScratchPad << :begin
|
|
x = true
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
def self.loop_next(arg)
|
|
x = 1
|
|
loop do
|
|
break if x == 2
|
|
|
|
begin
|
|
ScratchPad << :begin
|
|
x += 1
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
|
|
def self.loop_within_iter(arg)
|
|
yielding do
|
|
x = 1
|
|
loop do
|
|
break if x == 2
|
|
|
|
begin
|
|
ScratchPad << :begin
|
|
x += 1
|
|
if arg
|
|
next 42
|
|
else
|
|
next
|
|
end
|
|
ensure
|
|
ScratchPad << :ensure
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
class Block
|
|
def method(v)
|
|
yield v
|
|
end
|
|
end
|
|
end
|