mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
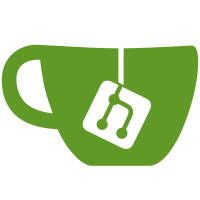
This reverts commits:10d6a3aca7
8ba48c1b85
fba8627dc1
dd883de5ba
6c6a25feca
167e6b48f1
7cb96d41a5
3207979278
595b3c4fdd
1521f7cf89
c11c5e69ac
cf33608203
3632a812c0
f56506be0d
86427a3219
. The reason for the revert is that we observe ABA problem around inline method cache. When a cache misshits, we search for a method entry. And if the entry is identical to what was cached before, we reuse the cache. But the commits we are reverting here introduced situations where a method entry is freed, then the identical memory region is used for another method entry. An inline method cache cannot detect that ABA. Here is a code that reproduce such situation: ```ruby require 'prime' class << Integer alias org_sqrt sqrt def sqrt(n) raise end GC.stress = true Prime.each(7*37){} rescue nil # <- Here we populate CC class << Object.new; end # These adjacent remove-then-alias maneuver # frees a method entry, then immediately # reuses it for another. remove_method :sqrt alias sqrt org_sqrt end Prime.each(7*37).to_a # <- SEGV ```
34 lines
1.5 KiB
C
34 lines
1.5 KiB
C
#ifndef RUBY_ID_TABLE_H
|
|
#define RUBY_ID_TABLE_H 1
|
|
#include "ruby/ruby.h"
|
|
|
|
struct rb_id_table;
|
|
|
|
/* compatible with ST_* */
|
|
enum rb_id_table_iterator_result {
|
|
ID_TABLE_CONTINUE = ST_CONTINUE,
|
|
ID_TABLE_STOP = ST_STOP,
|
|
ID_TABLE_DELETE = ST_DELETE,
|
|
ID_TABLE_REPLACE = ST_REPLACE,
|
|
ID_TABLE_ITERATOR_RESULT_END
|
|
};
|
|
|
|
struct rb_id_table *rb_id_table_create(size_t size);
|
|
void rb_id_table_free(struct rb_id_table *tbl);
|
|
void rb_id_table_clear(struct rb_id_table *tbl);
|
|
|
|
size_t rb_id_table_size(const struct rb_id_table *tbl);
|
|
size_t rb_id_table_memsize(const struct rb_id_table *tbl);
|
|
|
|
int rb_id_table_insert(struct rb_id_table *tbl, ID id, VALUE val);
|
|
int rb_id_table_lookup(struct rb_id_table *tbl, ID id, VALUE *valp);
|
|
int rb_id_table_delete(struct rb_id_table *tbl, ID id);
|
|
|
|
typedef enum rb_id_table_iterator_result rb_id_table_update_callback_func_t(ID *id, VALUE *val, void *data, int existing);
|
|
typedef enum rb_id_table_iterator_result rb_id_table_foreach_func_t(ID id, VALUE val, void *data);
|
|
typedef enum rb_id_table_iterator_result rb_id_table_foreach_values_func_t(VALUE val, void *data);
|
|
void rb_id_table_foreach(struct rb_id_table *tbl, rb_id_table_foreach_func_t *func, void *data);
|
|
void rb_id_table_foreach_with_replace(struct rb_id_table *tbl, rb_id_table_foreach_func_t *func, rb_id_table_update_callback_func_t *replace, void *data);
|
|
void rb_id_table_foreach_values(struct rb_id_table *tbl, rb_id_table_foreach_values_func_t *func, void *data);
|
|
|
|
#endif /* RUBY_ID_TABLE_H */
|