mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
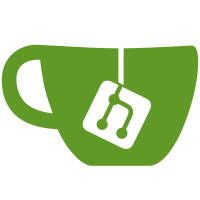
== SOAP client and server == === for both client side and server side === * improved document/literal service support. style(rpc,document)/use(encoding, literal) combination are all supported. for the detail about combination, see test/soap/test_style.rb. * let WSDLEncodedRegistry#soap2obj map SOAP/OM to Ruby according to WSDL as well as obj2soap. closes #70. * let SOAP::Mapping::Object handle XML attribute for doc/lit service. you can set/get XML attribute via accessor methods which as a name 'xmlattr_' prefixed (<foo name="bar"/> -> Foo#xmlattr_name). === client side === * WSDLDriver capitalized name operation bug fixed. from 1.5.3-ruby1.8.2, operation which has capitalized name (such as KeywordSearchRequest in AWS) is defined as a method having uncapitalized name. (converted with GenSupport.safemethodname to handle operation name 'foo-bar'). it introduced serious incompatibility; in the past, it was defined as a capitalized. define capitalized method as well under that circumstance. * added new factory interface 'WSDLDriverFactory#create_rpc_driver' to create RPC::Driver, not WSDLDriver (RPC::Driver and WSDLDriver are merged). 'WSDLDriverFactory#create_driver' still creates WSDLDriver for compatibility but it warns that the method is deprecated. please use create_rpc_driver instead of create_driver. * allow to use an URI object as an endpoint_url even with net/http, not http-access2. === server side === * added mod_ruby support to SOAP::CGIStub. rename a CGI script server.cgi to server.rb and let mod_ruby's RubyHandler handles the script. CGIStub detects if it's running under mod_ruby environment or not. * added fcgi support to SOAP::CGIStub. see the sample at sample/soap/calc/server.fcgi. (almost same as server.cgi but has fcgi handler at the bottom.) * allow to return a SOAPFault object to respond customized SOAP fault. * added the interface 'generate_explicit_type' for server side (CGIStub, HTTPServer). call 'self.generate_explicit_type = true' if you want to return simplified XML even if it's rpc/encoded service. == WSDL == === WSDL definition === * improved XML Schema support such as extension, restriction, simpleType, complexType + simpleContent, ref, length, import, include. * reduced "unknown element/attribute" warnings (warn only 1 time for each QName). * importing XSD file at schemaLocation with xsd:import. === code generation from WSDL === * generator crashed when there's '-' in defined element/attribute name. * added ApacheMap WSDL definition. * sample/{soap,wsdl}: removed. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@8500 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
269 lines
7.5 KiB
Ruby
269 lines
7.5 KiB
Ruby
# SOAP4R - WSDL encoded mapping registry.
|
|
# Copyright (C) 2000-2003, 2005 NAKAMURA, Hiroshi <nahi@ruby-lang.org>.
|
|
|
|
# This program is copyrighted free software by NAKAMURA, Hiroshi. You can
|
|
# redistribute it and/or modify it under the same terms of Ruby's license;
|
|
# either the dual license version in 2003, or any later version.
|
|
|
|
|
|
require 'xsd/qname'
|
|
require 'xsd/namedelements'
|
|
require 'soap/baseData'
|
|
require 'soap/mapping/mapping'
|
|
require 'soap/mapping/typeMap'
|
|
|
|
|
|
module SOAP
|
|
module Mapping
|
|
|
|
|
|
class WSDLEncodedRegistry < Registry
|
|
include TraverseSupport
|
|
|
|
attr_reader :definedelements
|
|
attr_reader :definedtypes
|
|
attr_accessor :excn_handler_obj2soap
|
|
attr_accessor :excn_handler_soap2obj
|
|
|
|
def initialize(definedtypes = XSD::NamedElements::Empty)
|
|
@definedtypes = definedtypes
|
|
# @definedelements = definedelements needed?
|
|
@excn_handler_obj2soap = nil
|
|
@excn_handler_soap2obj = nil
|
|
# For mapping AnyType element.
|
|
@rubytype_factory = RubytypeFactory.new(
|
|
:allow_untyped_struct => true,
|
|
:allow_original_mapping => true
|
|
)
|
|
@schema_element_cache = {}
|
|
end
|
|
|
|
def obj2soap(obj, qname = nil)
|
|
soap_obj = nil
|
|
if type = @definedtypes[qname]
|
|
soap_obj = obj2typesoap(obj, type)
|
|
else
|
|
soap_obj = any2soap(obj, qname)
|
|
end
|
|
return soap_obj if soap_obj
|
|
if @excn_handler_obj2soap
|
|
soap_obj = @excn_handler_obj2soap.call(obj) { |yield_obj|
|
|
Mapping._obj2soap(yield_obj, self)
|
|
}
|
|
return soap_obj if soap_obj
|
|
end
|
|
if qname
|
|
raise MappingError.new("cannot map #{obj.class.name} as #{qname}")
|
|
else
|
|
raise MappingError.new("cannot map #{obj.class.name} to SOAP/OM")
|
|
end
|
|
end
|
|
|
|
# map anything for now: must refer WSDL while mapping. [ToDo]
|
|
def soap2obj(node, obj_class = nil)
|
|
begin
|
|
return any2obj(node, obj_class)
|
|
rescue MappingError
|
|
end
|
|
if @excn_handler_soap2obj
|
|
begin
|
|
return @excn_handler_soap2obj.call(node) { |yield_node|
|
|
Mapping._soap2obj(yield_node, self)
|
|
}
|
|
rescue Exception
|
|
end
|
|
end
|
|
raise MappingError.new("cannot map #{node.type.name} to Ruby object")
|
|
end
|
|
|
|
private
|
|
|
|
def any2soap(obj, qname)
|
|
if obj.nil?
|
|
SOAPNil.new
|
|
elsif qname.nil? or qname == XSD::AnyTypeName
|
|
@rubytype_factory.obj2soap(nil, obj, nil, self)
|
|
elsif obj.is_a?(XSD::NSDBase)
|
|
soap2soap(obj, qname)
|
|
elsif (type = TypeMap[qname])
|
|
base2soap(obj, type)
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
|
|
def soap2soap(obj, type_qname)
|
|
if obj.is_a?(SOAPBasetype)
|
|
obj
|
|
elsif obj.is_a?(SOAPStruct) && (type = @definedtypes[type_qname])
|
|
soap_obj = obj
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
elements2soap(obj, soap_obj, type.content.elements)
|
|
soap_obj
|
|
elsif obj.is_a?(SOAPArray) && (type = @definedtypes[type_qname])
|
|
soap_obj = obj
|
|
contenttype = type.child_type
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
obj.replace do |ele|
|
|
Mapping._obj2soap(ele, self, contenttype)
|
|
end
|
|
soap_obj
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
|
|
def obj2typesoap(obj, type)
|
|
if type.is_a?(::WSDL::XMLSchema::SimpleType)
|
|
simpleobj2soap(obj, type)
|
|
else
|
|
complexobj2soap(obj, type)
|
|
end
|
|
end
|
|
|
|
def simpleobj2soap(obj, type)
|
|
type.check_lexical_format(obj)
|
|
return SOAPNil.new if obj.nil? # ToDo: check nillable.
|
|
o = base2soap(obj, TypeMap[type.base])
|
|
o
|
|
end
|
|
|
|
def complexobj2soap(obj, type)
|
|
case type.compoundtype
|
|
when :TYPE_STRUCT
|
|
struct2soap(obj, type.name, type)
|
|
when :TYPE_ARRAY
|
|
array2soap(obj, type.name, type)
|
|
when :TYPE_MAP
|
|
map2soap(obj, type.name, type)
|
|
when :TYPE_SIMPLE
|
|
simpleobj2soap(obj, type.simplecontent)
|
|
when :TYPE_EMPTY
|
|
raise MappingError.new("should be empty") unless obj.nil?
|
|
SOAPNil.new
|
|
else
|
|
raise MappingError.new("unknown compound type: #{type.compoundtype}")
|
|
end
|
|
end
|
|
|
|
def base2soap(obj, type)
|
|
soap_obj = nil
|
|
if type <= XSD::XSDString
|
|
soap_obj = type.new(XSD::Charset.is_ces(obj, $KCODE) ?
|
|
XSD::Charset.encoding_conv(obj, $KCODE, XSD::Charset.encoding) : obj)
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
else
|
|
soap_obj = type.new(obj)
|
|
end
|
|
soap_obj
|
|
end
|
|
|
|
def struct2soap(obj, type_qname, type)
|
|
return SOAPNil.new if obj.nil? # ToDo: check nillable.
|
|
soap_obj = SOAPStruct.new(type_qname)
|
|
unless obj.nil?
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
elements2soap(obj, soap_obj, type.content.elements)
|
|
end
|
|
soap_obj
|
|
end
|
|
|
|
def array2soap(obj, type_qname, type)
|
|
return SOAPNil.new if obj.nil? # ToDo: check nillable.
|
|
arytype = type.child_type
|
|
soap_obj = SOAPArray.new(ValueArrayName, 1, arytype)
|
|
unless obj.nil?
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
obj.each do |item|
|
|
soap_obj.add(Mapping._obj2soap(item, self, arytype))
|
|
end
|
|
end
|
|
soap_obj
|
|
end
|
|
|
|
MapKeyName = XSD::QName.new(nil, "key")
|
|
MapValueName = XSD::QName.new(nil, "value")
|
|
def map2soap(obj, type_qname, type)
|
|
return SOAPNil.new if obj.nil? # ToDo: check nillable.
|
|
keytype = type.child_type(MapKeyName) || XSD::AnyTypeName
|
|
valuetype = type.child_type(MapValueName) || XSD::AnyTypeName
|
|
soap_obj = SOAPStruct.new(MapQName)
|
|
unless obj.nil?
|
|
mark_marshalled_obj(obj, soap_obj)
|
|
obj.each do |key, value|
|
|
elem = SOAPStruct.new
|
|
elem.add("key", Mapping._obj2soap(key, self, keytype))
|
|
elem.add("value", Mapping._obj2soap(value, self, valuetype))
|
|
# ApacheAxis allows only 'item' here.
|
|
soap_obj.add("item", elem)
|
|
end
|
|
end
|
|
soap_obj
|
|
end
|
|
|
|
def elements2soap(obj, soap_obj, elements)
|
|
elements.each do |element|
|
|
name = element.name.name
|
|
child_obj = Mapping.get_attribute(obj, name)
|
|
soap_obj.add(name,
|
|
Mapping._obj2soap(child_obj, self, element.type || element.name))
|
|
end
|
|
end
|
|
|
|
def any2obj(node, obj_class)
|
|
unless obj_class
|
|
typestr = XSD::CodeGen::GenSupport.safeconstname(node.elename.name)
|
|
obj_class = Mapping.class_from_name(typestr)
|
|
end
|
|
if obj_class and obj_class.class_variables.include?('@@schema_element')
|
|
soap2stubobj(node, obj_class)
|
|
else
|
|
Mapping._soap2obj(node, Mapping::DefaultRegistry, obj_class)
|
|
end
|
|
end
|
|
|
|
def soap2stubobj(node, obj_class)
|
|
obj = Mapping.create_empty_object(obj_class)
|
|
unless node.is_a?(SOAPNil)
|
|
add_elements2stubobj(node, obj)
|
|
end
|
|
obj
|
|
end
|
|
|
|
def add_elements2stubobj(node, obj)
|
|
elements, as_array = schema_element_definition(obj.class)
|
|
vars = {}
|
|
node.each do |name, value|
|
|
if class_name = elements[name]
|
|
if klass = Mapping.class_from_name(class_name)
|
|
# klass must be a SOAPBasetype or a class
|
|
if klass.ancestors.include?(::SOAP::SOAPBasetype)
|
|
if value.respond_to?(:data)
|
|
child = klass.new(value.data).data
|
|
else
|
|
child = klass.new(nil).data
|
|
end
|
|
else
|
|
child = Mapping._soap2obj(value, self, klass)
|
|
end
|
|
else
|
|
raise MappingError.new("unknown class: #{class_name}")
|
|
end
|
|
else # untyped element is treated as anyType.
|
|
child = Mapping._soap2obj(value, self)
|
|
end
|
|
vars[name] = child
|
|
end
|
|
Mapping.set_attributes(obj, vars)
|
|
end
|
|
|
|
# it caches @@schema_element. this means that @@schema_element must not be
|
|
# changed while a lifetime of a WSDLLiteralRegistry.
|
|
def schema_element_definition(klass)
|
|
@schema_element_cache[klass] ||= Mapping.schema_element_definition(klass)
|
|
end
|
|
end
|
|
|
|
|
|
end
|
|
end
|