mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
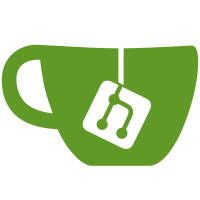
* win32/win32.c (rb_w32_write): writing to closed pipe fails with ERROR_NO_DATA but msvcrt maps it to EINVAL. map it to EPIPE. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@62150 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
49 lines
913 B
Ruby
49 lines
913 B
Ruby
# frozen_string_literal: false
|
|
require 'test/unit'
|
|
require_relative 'ut_eof'
|
|
|
|
class TestPipe < Test::Unit::TestCase
|
|
include TestEOF
|
|
def open_file(content)
|
|
r, w = IO.pipe
|
|
w << content
|
|
w.close
|
|
begin
|
|
yield r
|
|
ensure
|
|
r.close
|
|
end
|
|
end
|
|
class WithConversion < self
|
|
def open_file(content)
|
|
r, w = IO.pipe
|
|
w << content
|
|
w.close
|
|
r.set_encoding("us-ascii:utf-8")
|
|
begin
|
|
yield r
|
|
ensure
|
|
r.close
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_stdout_epipe
|
|
assert_separately([], "#{<<~"begin;"}\n#{<<~'end;'}")
|
|
begin;
|
|
io = STDOUT
|
|
begin
|
|
save = io.dup
|
|
IO.popen("echo", "w", out: IO::NULL) do |f|
|
|
io.reopen(f)
|
|
Process.wait(f.pid)
|
|
assert_raise(Errno::EPIPE) do
|
|
io.print "foo\n"
|
|
end
|
|
end
|
|
ensure
|
|
io.reopen(save)
|
|
end
|
|
end;
|
|
end
|
|
end
|