mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
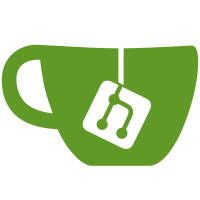
* For easier modifications of ruby/spec by MRI developers. * .gitignore: track changes under spec. * spec/mspec, spec/rubyspec: add in-tree mspec and ruby/spec. These files can therefore be updated like any other file in MRI. Instructions are provided in spec/README. [Feature #13156] [ruby-core:79246] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@58595 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
61 lines
1.3 KiB
Ruby
61 lines
1.3 KiB
Ruby
describe :thread_wakeup, shared: true do
|
|
it "can interrupt Kernel#sleep" do
|
|
exit_loop = false
|
|
after_sleep1 = false
|
|
after_sleep2 = false
|
|
|
|
t = Thread.new do
|
|
while true
|
|
break if exit_loop == true
|
|
Thread.pass
|
|
end
|
|
|
|
sleep
|
|
after_sleep1 = true
|
|
|
|
sleep
|
|
after_sleep2 = true
|
|
end
|
|
|
|
10.times { t.send(@method); Thread.pass }
|
|
t.status.should_not == "sleep"
|
|
|
|
exit_loop = true
|
|
|
|
10.times { sleep 0.1 if t.status and t.status != "sleep" }
|
|
after_sleep1.should == false # t should be blocked on the first sleep
|
|
t.send(@method)
|
|
|
|
10.times { sleep 0.1 if after_sleep1 != true }
|
|
10.times { sleep 0.1 if t.status and t.status != "sleep" }
|
|
after_sleep2.should == false # t should be blocked on the second sleep
|
|
t.send(@method)
|
|
|
|
t.join
|
|
end
|
|
|
|
it "does not result in a deadlock" do
|
|
t = Thread.new do
|
|
100.times { Thread.stop }
|
|
end
|
|
|
|
while t.status
|
|
begin
|
|
t.send(@method)
|
|
rescue ThreadError
|
|
# The thread might die right after.
|
|
t.status.should == false
|
|
end
|
|
Thread.pass
|
|
end
|
|
|
|
t.status.should == false
|
|
t.join
|
|
end
|
|
|
|
it "raises a ThreadError when trying to wake up a dead thread" do
|
|
t = Thread.new { 1 }
|
|
t.join
|
|
lambda { t.send @method }.should raise_error(ThreadError)
|
|
end
|
|
end
|