mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
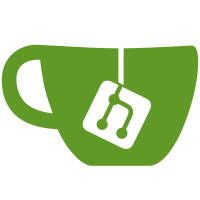
rubygems 2.7.x depends bundler-1.15.x. This is preparation for rubygems and bundler migration. * lib/bundler.rb, lib/bundler/*: files of bundler-1.15.4 * spec/bundler/*: rspec examples of bundler-1.15.4. I applied patches. * https://github.com/bundler/bundler/pull/6007 * Exclude not working examples on ruby repository. * Fake ruby interpriter instead of installed ruby. * Makefile.in: Added test task named `test-bundler`. This task is only working macOS/linux yet. I'm going to support Windows environment later. * tool/sync_default_gems.rb: Added sync task for bundler. [Feature #12733][ruby-core:77172] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@59779 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
110 lines
3.4 KiB
Ruby
110 lines
3.4 KiB
Ruby
# frozen_string_literal: true
|
|
require "spec_helper"
|
|
|
|
RSpec.describe "bundle install" do
|
|
describe "when a gem has a YAML gemspec" do
|
|
before :each do
|
|
build_repo2 do
|
|
build_gem "yaml_spec", :gemspec => :yaml
|
|
end
|
|
end
|
|
|
|
it "still installs correctly" do
|
|
gemfile <<-G
|
|
source "file://#{gem_repo2}"
|
|
gem "yaml_spec"
|
|
G
|
|
bundle :install
|
|
expect(err).to lack_errors
|
|
end
|
|
|
|
it "still installs correctly when using path" do
|
|
build_lib "yaml_spec", :gemspec => :yaml
|
|
|
|
install_gemfile <<-G
|
|
gem 'yaml_spec', :path => "#{lib_path("yaml_spec-1.0")}"
|
|
G
|
|
expect(err).to lack_errors
|
|
end
|
|
end
|
|
|
|
it "should use gemspecs in the system cache when available" do
|
|
gemfile <<-G
|
|
source "http://localtestserver.gem"
|
|
gem 'rack'
|
|
G
|
|
|
|
FileUtils.mkdir_p "#{tmp}/gems/system/specifications"
|
|
File.open("#{tmp}/gems/system/specifications/rack-1.0.0.gemspec", "w+") do |f|
|
|
spec = Gem::Specification.new do |s|
|
|
s.name = "rack"
|
|
s.version = "1.0.0"
|
|
s.add_runtime_dependency "activesupport", "2.3.2"
|
|
end
|
|
f.write spec.to_ruby
|
|
end
|
|
bundle :install, :artifice => "endpoint_marshal_fail" # force gemspec load
|
|
expect(the_bundle).to include_gems "activesupport 2.3.2"
|
|
end
|
|
|
|
context "when ruby version is specified in gemspec and gemfile" do
|
|
it "installs when patch level is not specified and the version matches" do
|
|
build_lib("foo", :path => bundled_app) do |s|
|
|
s.required_ruby_version = "~> #{RUBY_VERSION}.0"
|
|
end
|
|
|
|
install_gemfile <<-G
|
|
ruby '#{RUBY_VERSION}', :engine_version => '#{RUBY_VERSION}', :engine => 'ruby'
|
|
gemspec
|
|
G
|
|
expect(the_bundle).to include_gems "foo 1.0"
|
|
end
|
|
|
|
it "installs when patch level is specified and the version still matches the current version",
|
|
:if => RUBY_PATCHLEVEL >= 0 do
|
|
build_lib("foo", :path => bundled_app) do |s|
|
|
s.required_ruby_version = "#{RUBY_VERSION}.#{RUBY_PATCHLEVEL}"
|
|
end
|
|
|
|
install_gemfile <<-G
|
|
ruby '#{RUBY_VERSION}', :engine_version => '#{RUBY_VERSION}', :engine => 'ruby', :patchlevel => '#{RUBY_PATCHLEVEL}'
|
|
gemspec
|
|
G
|
|
expect(the_bundle).to include_gems "foo 1.0"
|
|
end
|
|
|
|
it "fails and complains about patchlevel on patchlevel mismatch",
|
|
:if => RUBY_PATCHLEVEL >= 0 do
|
|
patchlevel = RUBY_PATCHLEVEL.to_i + 1
|
|
build_lib("foo", :path => bundled_app) do |s|
|
|
s.required_ruby_version = "#{RUBY_VERSION}.#{patchlevel}"
|
|
end
|
|
|
|
install_gemfile <<-G
|
|
ruby '#{RUBY_VERSION}', :engine_version => '#{RUBY_VERSION}', :engine => 'ruby', :patchlevel => '#{patchlevel}'
|
|
gemspec
|
|
G
|
|
|
|
expect(out).to include("Ruby patchlevel")
|
|
expect(out).to include("but your Gemfile specified")
|
|
expect(exitstatus).to eq(18) if exitstatus
|
|
end
|
|
|
|
it "fails and complains about version on version mismatch" do
|
|
version = Gem::Requirement.create(RUBY_VERSION).requirements.first.last.bump.version
|
|
|
|
build_lib("foo", :path => bundled_app) do |s|
|
|
s.required_ruby_version = version
|
|
end
|
|
|
|
install_gemfile <<-G
|
|
ruby '#{version}', :engine_version => '#{version}', :engine => 'ruby'
|
|
gemspec
|
|
G
|
|
|
|
expect(out).to include("Ruby version")
|
|
expect(out).to include("but your Gemfile specified")
|
|
expect(exitstatus).to eq(18) if exitstatus
|
|
end
|
|
end
|
|
end
|