mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
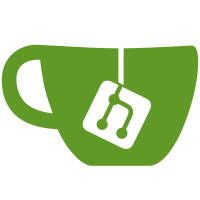
* Other ruby implementations use the spec/ruby directory. [Misc #13792] [ruby-core:82287] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@59979 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
68 lines
1,008 B
Ruby
68 lines
1,008 B
Ruby
module HashSpecs
|
|
class MyHash < Hash; end
|
|
|
|
class MyInitializerHash < Hash
|
|
|
|
def initialize
|
|
raise "Constructor called"
|
|
end
|
|
|
|
end
|
|
|
|
class NewHash < Hash
|
|
def initialize(*args)
|
|
args.each_with_index do |val, index|
|
|
self[index] = val
|
|
end
|
|
end
|
|
end
|
|
|
|
class DefaultHash < Hash
|
|
def default(key)
|
|
100
|
|
end
|
|
end
|
|
|
|
class ToHashHash < Hash
|
|
def to_hash
|
|
{ "to_hash" => "was", "called!" => "duh." }
|
|
end
|
|
end
|
|
|
|
class KeyWithPrivateHash
|
|
private :hash
|
|
end
|
|
|
|
class ByIdentityKey
|
|
def hash
|
|
fail("#hash should not be called on compare_by_identity Hash")
|
|
end
|
|
end
|
|
|
|
class ByValueKey
|
|
attr_reader :n
|
|
def initialize(n)
|
|
@n = n
|
|
end
|
|
|
|
def hash
|
|
n
|
|
end
|
|
|
|
def eql? other
|
|
ByValueKey === other and @n == other.n
|
|
end
|
|
end
|
|
|
|
def self.empty_frozen_hash
|
|
@empty ||= {}
|
|
@empty.freeze
|
|
@empty
|
|
end
|
|
|
|
def self.frozen_hash
|
|
@hash ||= { 1 => 2, 3 => 4 }
|
|
@hash.freeze
|
|
@hash
|
|
end
|
|
end
|