mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
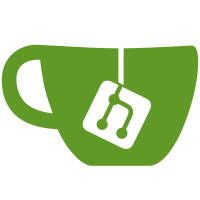
* Other ruby implementations use the spec/ruby directory. [Misc #13792] [ruby-core:82287] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@59979 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
67 lines
2.3 KiB
Ruby
67 lines
2.3 KiB
Ruby
require File.expand_path('../../../spec_helper', __FILE__)
|
|
require File.expand_path('../fixtures/classes', __FILE__)
|
|
|
|
describe "Numeric#remainder" do
|
|
before :each do
|
|
@obj = NumericSpecs::Subclass.new
|
|
@result = mock("Numeric#% result")
|
|
@other = mock("Passed Object")
|
|
end
|
|
|
|
it "returns the result of calling self#% with other if self is 0" do
|
|
@obj.should_receive(:%).with(@other).and_return(@result)
|
|
@result.should_receive(:==).with(0).and_return(true)
|
|
|
|
@obj.remainder(@other).should equal(@result)
|
|
end
|
|
|
|
it "returns the result of calling self#% with other if self and other are greater than 0" do
|
|
@obj.should_receive(:%).with(@other).and_return(@result)
|
|
@result.should_receive(:==).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:<).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:>).with(0).and_return(true)
|
|
@other.should_receive(:<).with(0).and_return(false)
|
|
|
|
@obj.remainder(@other).should equal(@result)
|
|
end
|
|
|
|
it "returns the result of calling self#% with other if self and other are less than 0" do
|
|
@obj.should_receive(:%).with(@other).and_return(@result)
|
|
@result.should_receive(:==).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:<).with(0).and_return(true)
|
|
@other.should_receive(:>).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:>).with(0).and_return(false)
|
|
|
|
@obj.remainder(@other).should equal(@result)
|
|
end
|
|
|
|
it "returns the result of calling self#% with other - other if self is greater than 0 and other is less than 0" do
|
|
@obj.should_receive(:%).with(@other).and_return(@result)
|
|
@result.should_receive(:==).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:<).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:>).with(0).and_return(true)
|
|
@other.should_receive(:<).with(0).and_return(true)
|
|
|
|
@result.should_receive(:-).with(@other).and_return(:result)
|
|
|
|
@obj.remainder(@other).should == :result
|
|
end
|
|
|
|
it "returns the result of calling self#% with other - other if self is less than 0 and other is greater than 0" do
|
|
@obj.should_receive(:%).with(@other).and_return(@result)
|
|
@result.should_receive(:==).with(0).and_return(false)
|
|
|
|
@obj.should_receive(:<).with(0).and_return(true)
|
|
@other.should_receive(:>).with(0).and_return(true)
|
|
|
|
@result.should_receive(:-).with(@other).and_return(:result)
|
|
|
|
@obj.remainder(@other).should == :result
|
|
end
|
|
end
|