mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
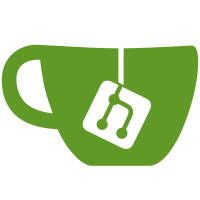
Using Fiber#transfer with Fiber#resume for a same Fiber is limited (once Fiber#transfer is called for a fiber, the fiber can not be resumed more). This restriction was introduced to protect the resume/yield chain, but we realized that it is too much to protect the chain. Instead of the current restriction, we introduce some other protections. (1) can not transfer to the resuming fiber. (2) can not transfer to the yielding fiber. (3) can not resume transferred fiber. (4) can not yield from not-resumed fiber. [Bug #17221] Also at the end of a transferred fiber, it had continued on root fiber. However, if the root fiber resumed a fiber (and that fiber can resumed another fiber), this behavior also breaks the resume/yield chain. So at the end of a transferred fiber, switch to the edge of resume chain from root fiber. For example, root fiber resumed f1 and f1 resumed f2, transferred to f3 and f3 terminated, then continue from the fiber f2 (it was continued from root fiber without this patch).
79 lines
2.3 KiB
Ruby
79 lines
2.3 KiB
Ruby
require_relative '../../spec_helper'
|
|
require_relative '../../shared/fiber/resume'
|
|
|
|
describe "Fiber#resume" do
|
|
it_behaves_like :fiber_resume, :resume
|
|
end
|
|
|
|
describe "Fiber#resume" do
|
|
it "runs until Fiber.yield" do
|
|
obj = mock('obj')
|
|
obj.should_not_receive(:do)
|
|
fiber = Fiber.new { 1 + 2; Fiber.yield; obj.do }
|
|
fiber.resume
|
|
end
|
|
|
|
it "resumes from the last call to Fiber.yield on subsequent invocations" do
|
|
fiber = Fiber.new { Fiber.yield :first; :second }
|
|
fiber.resume.should == :first
|
|
fiber.resume.should == :second
|
|
end
|
|
|
|
it "sets the block parameters to its arguments on the first invocation" do
|
|
first = mock('first')
|
|
first.should_receive(:arg).with(:first).twice
|
|
|
|
fiber = Fiber.new { |arg| first.arg arg; Fiber.yield; first.arg arg; }
|
|
fiber.resume :first
|
|
fiber.resume :second
|
|
end
|
|
|
|
ruby_version_is '3.0' do
|
|
it "raises a FiberError if the Fiber tries to resume itself" do
|
|
fiber = Fiber.new { fiber.resume }
|
|
-> { fiber.resume }.should raise_error(FiberError, /current fiber/)
|
|
end
|
|
end
|
|
|
|
ruby_version_is '' ... '3.0' do
|
|
it "raises a FiberError if the Fiber tries to resume itself" do
|
|
fiber = Fiber.new { fiber.resume }
|
|
-> { fiber.resume }.should raise_error(FiberError, /double resume/)
|
|
end
|
|
end
|
|
|
|
it "returns control to the calling Fiber if called from one" do
|
|
fiber1 = Fiber.new { :fiber1 }
|
|
fiber2 = Fiber.new { fiber1.resume; :fiber2 }
|
|
fiber2.resume.should == :fiber2
|
|
end
|
|
|
|
# Redmine #595
|
|
it "executes the ensure clause" do
|
|
code = <<-RUBY
|
|
f = Fiber.new do
|
|
begin
|
|
Fiber.yield
|
|
ensure
|
|
puts "ensure executed"
|
|
end
|
|
end
|
|
|
|
# The apparent issue is that when Fiber.yield executes, control
|
|
# "leaves" the "ensure block" and so the ensure clause should run. But
|
|
# control really does NOT leave the ensure block when Fiber.yield
|
|
# executes. It merely pauses there. To require ensure to run when a
|
|
# Fiber is suspended then makes ensure-in-a-Fiber-context different
|
|
# than ensure-in-a-Thread-context and this would be very confusing.
|
|
f.resume
|
|
|
|
# When we execute the second #resume call, the ensure block DOES exit,
|
|
# the ensure clause runs.
|
|
f.resume
|
|
|
|
exit 0
|
|
RUBY
|
|
|
|
ruby_exe(code).should == "ensure executed\n"
|
|
end
|
|
end
|