mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
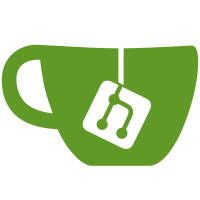
* For easier modifications of ruby/spec by MRI developers. * .gitignore: track changes under spec. * spec/mspec, spec/rubyspec: add in-tree mspec and ruby/spec. These files can therefore be updated like any other file in MRI. Instructions are provided in spec/README. [Feature #13156] [ruby-core:79246] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@58595 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
84 lines
2.2 KiB
Ruby
84 lines
2.2 KiB
Ruby
require File.expand_path('../../../../spec_helper', __FILE__)
|
|
require File.expand_path('../../fixtures/classes', __FILE__)
|
|
|
|
describe "BasicSocket#send" do
|
|
before :each do
|
|
@server = TCPServer.new('127.0.0.1', SocketSpecs.port)
|
|
@socket = TCPSocket.new('127.0.0.1', SocketSpecs.port)
|
|
end
|
|
|
|
after :each do
|
|
@server.closed?.should be_false
|
|
@socket.closed?.should be_false
|
|
|
|
@server.close
|
|
@socket.close
|
|
end
|
|
|
|
it "sends a message to another socket and returns the number of bytes sent" do
|
|
data = ""
|
|
t = Thread.new do
|
|
client = @server.accept
|
|
loop do
|
|
got = client.recv(5)
|
|
break if got.empty?
|
|
data << got
|
|
end
|
|
client.close
|
|
end
|
|
Thread.pass while t.status and t.status != "sleep"
|
|
t.status.should_not be_nil
|
|
|
|
@socket.send('hello', 0).should == 5
|
|
@socket.shutdown(1) # indicate, that we are done sending
|
|
@socket.recv(10)
|
|
|
|
t.join
|
|
data.should == 'hello'
|
|
end
|
|
|
|
platform_is_not :solaris, :windows do
|
|
it "accepts flags to specify unusual sending behaviour" do
|
|
data = nil
|
|
peek_data = nil
|
|
t = Thread.new do
|
|
client = @server.accept
|
|
peek_data = client.recv(6, Socket::MSG_PEEK)
|
|
data = client.recv(6)
|
|
client.recv(10) # this recv is important
|
|
client.close
|
|
end
|
|
Thread.pass while t.status and t.status != "sleep"
|
|
t.status.should_not be_nil
|
|
|
|
@socket.send('helloU', Socket::MSG_PEEK | Socket::MSG_OOB).should == 6
|
|
@socket.shutdown # indicate, that we are done sending
|
|
|
|
t.join
|
|
peek_data.should == "hello"
|
|
data.should == 'hello'
|
|
end
|
|
end
|
|
|
|
it "accepts a sockaddr as recipient address" do
|
|
data = ""
|
|
t = Thread.new do
|
|
client = @server.accept
|
|
loop do
|
|
got = client.recv(5)
|
|
break if got.empty?
|
|
data << got
|
|
end
|
|
client.close
|
|
end
|
|
Thread.pass while t.status and t.status != "sleep"
|
|
t.status.should_not be_nil
|
|
|
|
sockaddr = Socket.pack_sockaddr_in(SocketSpecs.port, "127.0.0.1")
|
|
@socket.send('hello', 0, sockaddr).should == 5
|
|
@socket.shutdown # indicate, that we are done sending
|
|
|
|
t.join
|
|
data.should == 'hello'
|
|
end
|
|
end
|