mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
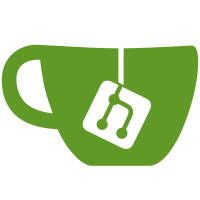
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@14161 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
80 lines
2.2 KiB
Ruby
80 lines
2.2 KiB
Ruby
require 'test/unit'
|
|
|
|
class TestRegexp < Test::Unit::TestCase
|
|
def test_ruby_dev_24643
|
|
assert_nothing_raised("[ruby-dev:24643]") { /(?:(?:[a]*[a])?b)*a*$/ =~ "aabaaca" }
|
|
end
|
|
|
|
def test_ruby_talk_116455
|
|
assert_match(/^(\w{2,}).* ([A-Za-z\xa2\xc0-\xff]{2,}?)$/, "Hallo Welt")
|
|
end
|
|
|
|
def test_ruby_dev_24887
|
|
assert_equal("a".gsub(/a\Z/, ""), "")
|
|
end
|
|
|
|
def test_yoshidam_net_20041111_1
|
|
s = "[\xC2\xA0-\xC3\xBE]"
|
|
assert_match(Regexp.new(s, nil, "u"), "\xC3\xBE")
|
|
end
|
|
|
|
def test_yoshidam_net_20041111_2
|
|
assert_raise(RegexpError) do
|
|
s = "[\xFF-\xFF]".force_encoding("utf-8")
|
|
Regexp.new(s, nil, "u")
|
|
end
|
|
end
|
|
|
|
def test_ruby_dev_31309
|
|
assert_equal('Ruby', 'Ruby'.sub(/[^a-z]/i, '-'))
|
|
end
|
|
|
|
def test_assert_normal_exit
|
|
# moved from knownbug. It caused core.
|
|
Regexp.union("a", "a")
|
|
end
|
|
|
|
def test_to_s
|
|
assert_equal '(?-mix:\000)', Regexp.new("\0").to_s
|
|
end
|
|
|
|
def test_union
|
|
assert_equal :ok, begin
|
|
Regexp.union(
|
|
"a",
|
|
Regexp.new("\xc2\xa1".force_encoding("euc-jp")),
|
|
Regexp.new("\xc2\xa1".force_encoding("utf-8")))
|
|
:ng
|
|
rescue ArgumentError
|
|
:ok
|
|
end
|
|
end
|
|
|
|
def test_named_capture
|
|
m = /&(?<foo>.*?);/.match("aaa & yyy")
|
|
assert_equal("amp", m["foo"])
|
|
assert_equal("amp", m[:foo])
|
|
assert_equal(5, m.begin(:foo))
|
|
assert_equal(8, m.end(:foo))
|
|
assert_equal([5,8], m.offset(:foo))
|
|
|
|
assert_equal("aaa [amp] yyy", "aaa & yyy".sub(/&(?<foo>.*?);/, '[\k<foo>]'))
|
|
|
|
assert_equal('#<MatchData "& y" foo:"amp">',
|
|
/&(?<foo>.*?); (y)/.match("aaa & yyy").inspect)
|
|
assert_equal('#<MatchData "& y" 1:"amp" 2:"y">',
|
|
/&(.*?); (y)/.match("aaa & yyy").inspect)
|
|
assert_equal('#<MatchData "& y" foo:"amp" bar:"y">',
|
|
/&(?<foo>.*?); (?<bar>y)/.match("aaa & yyy").inspect)
|
|
assert_equal('#<MatchData "& y" foo:"amp" foo:"y">',
|
|
/&(?<foo>.*?); (?<foo>y)/.match("aaa & yyy").inspect)
|
|
|
|
/(?<id>[A-Za-z_]+)/ =~ "!abc"
|
|
assert_equal("abc", Regexp.last_match(:id))
|
|
|
|
/a/ =~ "b"
|
|
assert_equal(nil, Regexp.last_match)
|
|
assert_equal(nil, Regexp.last_match(1))
|
|
assert_equal(nil, Regexp.last_match(:foo))
|
|
end
|
|
end
|