mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
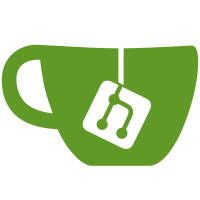
* tool/asm_parse.rb: add description * tool/change_maker.rb: ditto * tool/downloader.rb: ditto * tool/eval.rb: ditto * tool/expand-config.rb: ditto * tool/extlibs.rb: ditto * tool/fake.rb: ditto * tool/file2lastrev.rb: ditto * tool/gem-unpack.rb: ditto * tool/gen_dummy_probes.rb: ditto * tool/gen_ruby_tapset.rb: ditto * tool/generic_erb.rb: ditto * tool/id2token.rb: ditto * tool/ifchange: ditto * tool/insns2vm.rb: ditto * tool/instruction.rb: ditto * tool/jisx0208.rb: ditto * tool/merger.rb: ditto * tool/mkrunnable.rb: ditto * tool/node_name.rb: ditto * tool/parse.rb: ditto * tool/rbinstall.rb: ditto * tool/rbuninstall.rb: ditto * tool/rmdirs: ditto * tool/runruby.rb: ditto * tool/strip-rdoc.rb: ditto * tool/vcs.rb: ditto * tool/vtlh.rb: ditto * tool/ytab.sed: ditto * tool/enc-unicode.rb: fix typo * tool/mk_call_iseq_optimized.rb: ditto * tool/update-deps: ditto [ruby-core:76215] [Bug #12539] by Noah Gibbs <the.codefolio.guy@gmail.com> git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@55564 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
157 lines
3.6 KiB
Ruby
Executable file
157 lines
3.6 KiB
Ruby
Executable file
#!/usr/bin/ruby
|
|
|
|
# Used to download, extract and patch extension libraries (extlibs)
|
|
# for Ruby. See common.mk for Ruby's usage.
|
|
|
|
require 'fileutils'
|
|
require 'digest'
|
|
require_relative 'downloader'
|
|
|
|
def do_download(url, base, cache_dir)
|
|
Downloader.download(url, base, cache_dir, nil)
|
|
end
|
|
|
|
def do_checksum(cache, chksums)
|
|
chksums.each do |sum|
|
|
name, sum = sum.split(/:/)
|
|
if $VERBOSE
|
|
$stdout.print "checking #{name} of #{cache} ..."
|
|
$stdout.flush
|
|
end
|
|
hd = Digest(name.upcase).file(cache).hexdigest
|
|
if hd == sum
|
|
if $VERBOSE
|
|
$stdout.puts " OK"
|
|
$stdout.flush
|
|
end
|
|
else
|
|
if $VERBOSE
|
|
$stdout.puts " NG"
|
|
$stdout.flush
|
|
end
|
|
raise "checksum mismatch: #{cache}, #{name}:#{hd}, expected #{sum}"
|
|
end
|
|
end
|
|
end
|
|
|
|
def do_extract(cache, dir)
|
|
if $VERBOSE
|
|
$stdout.puts "extracting #{cache} into #{dir}"
|
|
$stdout.flush
|
|
end
|
|
ext = File.extname(cache)
|
|
case ext
|
|
when '.gz', '.tgz'
|
|
f = IO.popen(["gzip", "-dc", cache])
|
|
cache = cache.chomp('.gz')
|
|
when '.bz2', '.tbz'
|
|
f = IO.popen(["bzip2", "-dc", cache])
|
|
cache = cache.chomp('.bz2')
|
|
when '.xz', '.txz'
|
|
f = IO.popen(["xz", "-dc", cache])
|
|
cache = cache.chomp('.xz')
|
|
else
|
|
inp = cache
|
|
end
|
|
inp ||= f.binmode
|
|
ext = File.extname(cache)
|
|
case ext
|
|
when '.tar', /\A\.t[gbx]z\z/
|
|
pid = Process.spawn("tar", "xpf", "-", in: inp, chdir: dir)
|
|
when '.zip'
|
|
pid = Process.spawn("unzip", inp, "-d", dir)
|
|
end
|
|
f.close if f
|
|
Process.wait(pid)
|
|
$?.success? or raise "failed to extract #{cache}"
|
|
end
|
|
|
|
def do_patch(dest, patch, args)
|
|
if $VERBOSE
|
|
$stdout.puts "applying #{patch} under #{dest}"
|
|
$stdout.flush
|
|
end
|
|
Process.wait(Process.spawn("patch", "-d", dest, "-i", patch, *args))
|
|
$?.success? or raise "failed to patch #{patch}"
|
|
end
|
|
|
|
cache_dir = ENV['CACHE_DIR'] || ".downloaded-cache"
|
|
mode = :all
|
|
until ARGV.empty?
|
|
case ARGV[0]
|
|
when '--download'
|
|
mode = :download
|
|
when '--extract'
|
|
mode = :extract
|
|
when '--patch'
|
|
mode = :patch
|
|
when '--all'
|
|
mode = :all
|
|
when '--cache'
|
|
ARGV.shift
|
|
cache_dir = ARGV[0]
|
|
when /\A--cache=/
|
|
cache_dir = $'
|
|
when '--'
|
|
ARGV.shift
|
|
break
|
|
when /\A-/
|
|
abort "unknown option: #{ARGV[0]}"
|
|
else
|
|
break
|
|
end
|
|
ARGV.shift
|
|
end
|
|
|
|
FileUtils.mkdir_p(cache_dir)
|
|
|
|
success = true
|
|
ARGV.each do |dir|
|
|
Dir.glob("#{dir}/**/extlibs") do |list|
|
|
if $VERBOSE
|
|
$stdout.puts "downloading for #{list}"
|
|
$stdout.flush
|
|
end
|
|
extracted = false
|
|
dest = File.dirname(list)
|
|
IO.foreach(list) do |line|
|
|
line.sub!(/\s*#.*/, '')
|
|
if /^\t/ =~ line
|
|
if extracted and (mode == :all or mode == :patch)
|
|
patch, *args = line.split
|
|
do_patch(dest, patch, args)
|
|
end
|
|
next
|
|
end
|
|
url, *chksums = line.split(' ')
|
|
next unless url
|
|
extracted = false
|
|
base = File.basename(url)
|
|
cache = File.join(cache_dir, base)
|
|
target = File.join(dest, base[/.*(?=\.tar(?:\.\w+)?\z)/])
|
|
begin
|
|
case mode
|
|
when :download
|
|
do_download(url, base, cache_dir)
|
|
do_checksum(cache, chksums)
|
|
when :extract
|
|
unless File.directory?(target)
|
|
do_checksum(cache, chksums)
|
|
extracted = do_extract(cache, dest)
|
|
end
|
|
when :all
|
|
do_download(url, base, cache_dir)
|
|
unless File.directory?(target)
|
|
do_checksum(cache, chksums)
|
|
extracted = do_extract(cache, dest)
|
|
end
|
|
end
|
|
rescue => e
|
|
warn e.inspect
|
|
success = false
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
exit(success)
|