mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
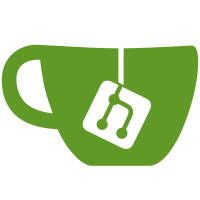
This removes the related tests, and puts the related specs behind version guards. This affects all code in lib, including some libraries that may want to support older versions of Ruby.
50 lines
1.4 KiB
Ruby
50 lines
1.4 KiB
Ruby
# encoding: utf-8
|
|
|
|
require_relative '../../spec_helper'
|
|
require_relative 'fixtures/classes'
|
|
|
|
describe "String#reverse" do
|
|
it "returns a new string with the characters of self in reverse order" do
|
|
"stressed".reverse.should == "desserts"
|
|
"m".reverse.should == "m"
|
|
"".reverse.should == ""
|
|
end
|
|
|
|
ruby_version_is ''...'2.7' do
|
|
it "taints the result if self is tainted" do
|
|
"".taint.reverse.tainted?.should == true
|
|
"m".taint.reverse.tainted?.should == true
|
|
end
|
|
end
|
|
|
|
it "reverses a string with multi byte characters" do
|
|
"微軟正黑體".reverse.should == "體黑正軟微"
|
|
end
|
|
|
|
end
|
|
|
|
describe "String#reverse!" do
|
|
it "reverses self in place and always returns self" do
|
|
a = "stressed"
|
|
a.reverse!.should equal(a)
|
|
a.should == "desserts"
|
|
|
|
"".reverse!.should == ""
|
|
end
|
|
|
|
it "raises a #{frozen_error_class} on a frozen instance that is modified" do
|
|
-> { "anna".freeze.reverse! }.should raise_error(frozen_error_class)
|
|
-> { "hello".freeze.reverse! }.should raise_error(frozen_error_class)
|
|
end
|
|
|
|
# see [ruby-core:23666]
|
|
it "raises a #{frozen_error_class} on a frozen instance that would not be modified" do
|
|
-> { "".freeze.reverse! }.should raise_error(frozen_error_class)
|
|
end
|
|
|
|
it "reverses a string with multi byte characters" do
|
|
str = "微軟正黑體"
|
|
str.reverse!
|
|
str.should == "體黑正軟微"
|
|
end
|
|
end
|