mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
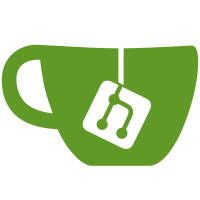
This removes the related tests, and puts the related specs behind version guards. This affects all code in lib, including some libraries that may want to support older versions of Ruby.
62 lines
1.7 KiB
Ruby
62 lines
1.7 KiB
Ruby
require_relative '../../spec_helper'
|
|
require_relative 'fixtures/classes'
|
|
|
|
describe "String#strip" do
|
|
it "returns a new string with leading and trailing whitespace removed" do
|
|
" hello ".strip.should == "hello"
|
|
" hello world ".strip.should == "hello world"
|
|
"\tgoodbye\r\v\n".strip.should == "goodbye"
|
|
"\x00 goodbye \x00".strip.should == "\x00 goodbye"
|
|
end
|
|
|
|
it "returns a copy of self with trailing NULL bytes and whitespace" do
|
|
" \x00 goodbye \x00 ".strip.should == "\x00 goodbye"
|
|
end
|
|
|
|
ruby_version_is ''...'2.7' do
|
|
it "taints the result when self is tainted" do
|
|
"".taint.strip.tainted?.should == true
|
|
"ok".taint.strip.tainted?.should == true
|
|
" ok ".taint.strip.tainted?.should == true
|
|
end
|
|
end
|
|
end
|
|
|
|
describe "String#strip!" do
|
|
it "modifies self in place and returns self" do
|
|
a = " hello "
|
|
a.strip!.should equal(a)
|
|
a.should == "hello"
|
|
|
|
a = "\tgoodbye\r\v\n"
|
|
a.strip!
|
|
a.should == "goodbye"
|
|
|
|
a = "\000 goodbye \000"
|
|
a.strip!
|
|
a.should == "\000 goodbye"
|
|
|
|
end
|
|
|
|
it "returns nil if no modifications where made" do
|
|
a = "hello"
|
|
a.strip!.should == nil
|
|
a.should == "hello"
|
|
end
|
|
|
|
it "modifies self removing trailing NULL bytes and whitespace" do
|
|
a = " \x00 goodbye \x00 "
|
|
a.strip!
|
|
a.should == "\x00 goodbye"
|
|
end
|
|
|
|
it "raises a #{frozen_error_class} on a frozen instance that is modified" do
|
|
-> { " hello ".freeze.strip! }.should raise_error(frozen_error_class)
|
|
end
|
|
|
|
# see #1552
|
|
it "raises a #{frozen_error_class} on a frozen instance that would not be modified" do
|
|
-> {"hello".freeze.strip! }.should raise_error(frozen_error_class)
|
|
-> {"".freeze.strip! }.should raise_error(frozen_error_class)
|
|
end
|
|
end
|