mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
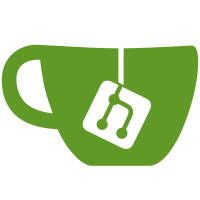
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@62656 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
74 lines
1.7 KiB
Ruby
74 lines
1.7 KiB
Ruby
require_relative '../spec_helper'
|
|
require_relative 'fixtures/match_operators'
|
|
|
|
describe "The !~ operator" do
|
|
before :each do
|
|
@obj = OperatorImplementor.new
|
|
end
|
|
|
|
it "evaluates as a call to !~" do
|
|
expected = "hello world"
|
|
|
|
opval = (@obj !~ expected)
|
|
methodval = @obj.send(:"!~", expected)
|
|
|
|
opval.should == expected
|
|
methodval.should == expected
|
|
end
|
|
end
|
|
|
|
describe "The =~ operator" do
|
|
before :each do
|
|
@impl = OperatorImplementor.new
|
|
end
|
|
|
|
it "calls the =~ method" do
|
|
expected = "hello world"
|
|
|
|
opval = (@obj =~ expected)
|
|
methodval = @obj.send(:"=~", expected)
|
|
|
|
opval.should == expected
|
|
methodval.should == expected
|
|
end
|
|
end
|
|
|
|
describe "The =~ operator with named captures" do
|
|
before :each do
|
|
@regexp = /(?<matched>foo)(?<unmatched>bar)?/
|
|
@string = "foofoo"
|
|
end
|
|
|
|
describe "on syntax of /regexp/ =~ string_variable" do
|
|
it "sets local variables by the captured pairs" do
|
|
/(?<matched>foo)(?<unmatched>bar)?/ =~ @string
|
|
local_variables.should == [:matched, :unmatched]
|
|
matched.should == "foo"
|
|
unmatched.should == nil
|
|
end
|
|
end
|
|
|
|
describe "on syntax of string_variable =~ /regexp/" do
|
|
it "does not set local variables" do
|
|
@string =~ /(?<matched>foo)(?<unmatched>bar)?/
|
|
local_variables.should == []
|
|
end
|
|
end
|
|
|
|
describe "on syntax of regexp_variable =~ string_variable" do
|
|
it "does not set local variables" do
|
|
@regexp =~ @string
|
|
local_variables.should == []
|
|
end
|
|
end
|
|
|
|
describe "on the method calling" do
|
|
it "does not set local variables" do
|
|
@regexp.=~(@string)
|
|
local_variables.should == []
|
|
|
|
@regexp.send :=~, @string
|
|
local_variables.should == []
|
|
end
|
|
end
|
|
end
|