mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
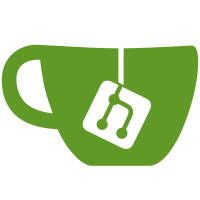
Previously, YJIT assumed that it's always possible to generate a new
basic block when servicing a stub in branch_stub_hit(). When YJIT is out
of executable memory, for example, this assumption doesn't hold up.
Add handling to branch_stub_hit() for servicing stubs without consuming
more executable memory by adding a code path that exits to the
interpreter at the location the branch stub represents. The new code
path reconstructs interpreter state in branch_stub_hit() and then exits
with a new snippet called `code_for_exit_from_stub` that returns
`Qundef` from the YJIT native stack frame.
As this change adds another place where we regenerate code from
`branch_t`, extract the logic for it into a new function and call it
regenerate_branch(). While we are at it, make the branch shrinking code
path in branch_stub_hit() more explicit.
This new functionality is hard to test without full support for out of
memory conditions. To verify this change, I ran
`RUBY_YJIT_ENABLE=1 make check -j12` with the following patch to stress
test the new code path:
```diff
diff --git a/yjit_core.c b/yjit_core.c
index 4ab63d9806..5788b8c5ed 100644
--- a/yjit_core.c
+++ b/yjit_core.c
@@ -878,8 +878,12 @@ branch_stub_hit(branch_t *branch, const uint32_t target_idx, rb_execution_contex
cb_set_write_ptr(cb, branch->end_addr);
}
+if (rand() < RAND_MAX/2) {
// Compile the new block version
p_block = gen_block_version(target, target_ctx, ec);
+}else{
+ p_block = NULL;
+}
if (!p_block && branch_modified) {
// We couldn't generate a new block for the branch, but we modified the branch.
```
We can enable the new test along with other OOM tests once full support
lands.
Other small changes:
* yjit_utils.c (print_str): Update to work with new native frame shape.
Follow up for 8fa0ee4d40
.
* yjit_iface.c (rb_yjit_init): Run yjit_init_core() after
yjit_init_codegen() so `cb` and `ocb` are available.
109 lines
2.1 KiB
C
109 lines
2.1 KiB
C
// This file is a fragment of the yjit.o compilation unit. See yjit.c.
|
|
|
|
// Save caller-save registers on the stack before a C call
|
|
static void
|
|
push_regs(codeblock_t *cb)
|
|
{
|
|
push(cb, RAX);
|
|
push(cb, RCX);
|
|
push(cb, RDX);
|
|
push(cb, RSI);
|
|
push(cb, RDI);
|
|
push(cb, R8);
|
|
push(cb, R9);
|
|
push(cb, R10);
|
|
push(cb, R11);
|
|
pushfq(cb);
|
|
}
|
|
|
|
// Restore caller-save registers from the after a C call
|
|
static void
|
|
pop_regs(codeblock_t *cb)
|
|
{
|
|
popfq(cb);
|
|
pop(cb, R11);
|
|
pop(cb, R10);
|
|
pop(cb, R9);
|
|
pop(cb, R8);
|
|
pop(cb, RDI);
|
|
pop(cb, RSI);
|
|
pop(cb, RDX);
|
|
pop(cb, RCX);
|
|
pop(cb, RAX);
|
|
}
|
|
|
|
static void
|
|
print_int_cfun(int64_t val)
|
|
{
|
|
fprintf(stderr, "%lld\n", (long long int)val);
|
|
}
|
|
|
|
RBIMPL_ATTR_MAYBE_UNUSED()
|
|
static void
|
|
print_int(codeblock_t *cb, x86opnd_t opnd)
|
|
{
|
|
push_regs(cb);
|
|
|
|
if (opnd.num_bits < 64 && opnd.type != OPND_IMM)
|
|
movsx(cb, RDI, opnd);
|
|
else
|
|
mov(cb, RDI, opnd);
|
|
|
|
// Call the print function
|
|
mov(cb, RAX, const_ptr_opnd((void*)&print_int_cfun));
|
|
call(cb, RAX);
|
|
|
|
pop_regs(cb);
|
|
}
|
|
|
|
static void
|
|
print_ptr_cfun(void *val)
|
|
{
|
|
fprintf(stderr, "%p\n", val);
|
|
}
|
|
|
|
RBIMPL_ATTR_MAYBE_UNUSED()
|
|
static void
|
|
print_ptr(codeblock_t *cb, x86opnd_t opnd)
|
|
{
|
|
assert (opnd.num_bits == 64);
|
|
|
|
push_regs(cb);
|
|
|
|
mov(cb, RDI, opnd);
|
|
mov(cb, RAX, const_ptr_opnd((void*)&print_ptr_cfun));
|
|
call(cb, RAX);
|
|
|
|
pop_regs(cb);
|
|
}
|
|
|
|
static void
|
|
print_str_cfun(const char *str)
|
|
{
|
|
fprintf(stderr, "%s\n", str);
|
|
}
|
|
|
|
// Print a constant string to stdout
|
|
static void
|
|
print_str(codeblock_t *cb, const char *str)
|
|
{
|
|
//as.comment("printStr(\"" ~ str ~ "\")");
|
|
size_t len = strlen(str);
|
|
|
|
push_regs(cb);
|
|
|
|
// Load the string address and jump over the string data
|
|
lea(cb, RDI, mem_opnd(8, RIP, 5));
|
|
jmp32(cb, (int32_t)len + 1);
|
|
|
|
// Write the string chars and a null terminator
|
|
for (size_t i = 0; i < len; ++i)
|
|
cb_write_byte(cb, (uint8_t)str[i]);
|
|
cb_write_byte(cb, 0);
|
|
|
|
// Call the print function
|
|
mov(cb, RAX, const_ptr_opnd((void*)&print_str_cfun));
|
|
call(cb, RAX);
|
|
|
|
pop_regs(cb);
|
|
}
|