mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
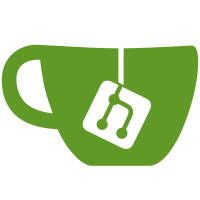
when $SAFE is set to 4. $SAFE=4 is now obsolete. [ruby-core:55222] [Feature #8468] * object.c (rb_obj_untrusted, rb_obj_untrust, rb_obj_trust): Kernel#untrusted?, untrust, and trust are now deprecated. Their behavior is same as tainted?, taint, and untaint, respectively. * include/ruby/ruby.h (OBJ_UNTRUSTED, OBJ_UNTRUST): OBJ_UNTRUSTED() and OBJ_UNTRUST() are aliases of OBJ_TAINTED() and OBJ_TAINT(), respectively. * array.c, class.c, debug.c, dir.c, encoding.c, error.c, eval.c, ext/curses/curses.c, ext/dbm/dbm.c, ext/dl/cfunc.c, ext/dl/cptr.c, ext/dl/dl.c, ext/etc/etc.c, ext/fiddle/fiddle.c, ext/fiddle/pointer.c, ext/gdbm/gdbm.c, ext/readline/readline.c, ext/sdbm/init.c, ext/socket/ancdata.c, ext/socket/basicsocket.c, ext/socket/socket.c, ext/socket/udpsocket.c, ext/stringio/stringio.c, ext/syslog/syslog.c, ext/tk/tcltklib.c, ext/win32ole/win32ole.c, file.c, gc.c, hash.c, io.c, iseq.c, load.c, marshal.c, object.c, proc.c, process.c, random.c, re.c, safe.c, string.c, thread.c, transcode.c, variable.c, vm_insnhelper.c, vm_method.c, vm_trace.c: remove code for $SAFE=4. * test/dl/test_dl2.rb, test/erb/test_erb.rb, test/readline/test_readline.rb, test/readline/test_readline_history.rb, test/ruby/test_alias.rb, test/ruby/test_array.rb, test/ruby/test_dir.rb, test/ruby/test_encoding.rb, test/ruby/test_env.rb, test/ruby/test_eval.rb, test/ruby/test_exception.rb, test/ruby/test_file_exhaustive.rb, test/ruby/test_hash.rb, test/ruby/test_io.rb, test/ruby/test_method.rb, test/ruby/test_module.rb, test/ruby/test_object.rb, test/ruby/test_pack.rb, test/ruby/test_rand.rb, test/ruby/test_regexp.rb, test/ruby/test_settracefunc.rb, test/ruby/test_struct.rb, test/ruby/test_thread.rb, test/ruby/test_time.rb: remove tests for $SAFE=4. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@41259 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
140 lines
3.7 KiB
Ruby
140 lines
3.7 KiB
Ruby
require_relative 'test_base.rb'
|
|
require 'dl/callback'
|
|
require 'dl/func'
|
|
require 'dl/pack'
|
|
|
|
module DL
|
|
class TestDL < TestBase
|
|
def ptr2num(*list)
|
|
list.pack("p*").unpack(PackInfo::PACK_MAP[TYPE_VOIDP] + "*")
|
|
end
|
|
|
|
# TODO: refactor test repetition
|
|
|
|
def test_realloc
|
|
str = "abc"
|
|
ptr_id = DL.realloc(0, 4)
|
|
ptr = CPtr.new(ptr_id, 4)
|
|
|
|
assert_equal ptr_id, ptr.to_i
|
|
|
|
cfunc = CFunc.new(@libc['strcpy'], TYPE_VOIDP, 'strcpy')
|
|
cfunc.call([ptr_id,str].pack("l!p").unpack("l!*"))
|
|
assert_equal("abc\0", ptr[0,4])
|
|
DL.free ptr_id
|
|
end
|
|
|
|
def test_malloc
|
|
str = "abc"
|
|
|
|
ptr_id = DL.malloc(4)
|
|
ptr = CPtr.new(ptr_id, 4)
|
|
|
|
assert_equal ptr_id, ptr.to_i
|
|
|
|
cfunc = CFunc.new(@libc['strcpy'], TYPE_VOIDP, 'strcpy')
|
|
cfunc.call([ptr_id,str].pack("l!p").unpack("l!*"))
|
|
assert_equal("abc\0", ptr[0,4])
|
|
DL.free ptr_id
|
|
end
|
|
|
|
def test_call_int()
|
|
cfunc = CFunc.new(@libc['atoi'], TYPE_INT, 'atoi')
|
|
x = cfunc.call(["100"].pack("p").unpack("l!*"))
|
|
assert_equal(100, x)
|
|
|
|
cfunc = CFunc.new(@libc['atoi'], TYPE_INT, 'atoi')
|
|
x = cfunc.call(["-100"].pack("p").unpack("l!*"))
|
|
assert_equal(-100, x)
|
|
end
|
|
|
|
def test_call_long()
|
|
cfunc = CFunc.new(@libc['atol'], TYPE_LONG, 'atol')
|
|
x = cfunc.call(["100"].pack("p").unpack("l!*"))
|
|
assert_equal(100, x)
|
|
cfunc = CFunc.new(@libc['atol'], TYPE_LONG, 'atol')
|
|
x = cfunc.call(["-100"].pack("p").unpack("l!*"))
|
|
assert_equal(-100, x)
|
|
end
|
|
|
|
def test_call_double()
|
|
cfunc = CFunc.new(@libc['atof'], TYPE_DOUBLE, 'atof')
|
|
x = cfunc.call(["0.1"].pack("p").unpack("l!*"))
|
|
assert_in_delta(0.1, x)
|
|
|
|
cfunc = CFunc.new(@libc['atof'], TYPE_DOUBLE, 'atof')
|
|
x = cfunc.call(["-0.1"].pack("p").unpack("l!*"))
|
|
assert_in_delta(-0.1, x)
|
|
end
|
|
|
|
def test_sin
|
|
return if /x86_64/ =~ RUBY_PLATFORM
|
|
pi_2 = Math::PI/2
|
|
cfunc = Function.new(CFunc.new(@libm['sin'], TYPE_DOUBLE, 'sin'),
|
|
[TYPE_DOUBLE])
|
|
x = cfunc.call(pi_2)
|
|
assert_equal(Math.sin(pi_2), x)
|
|
|
|
cfunc = Function.new(CFunc.new(@libm['sin'], TYPE_DOUBLE, 'sin'),
|
|
[TYPE_DOUBLE])
|
|
x = cfunc.call(-pi_2)
|
|
assert_equal(Math.sin(-pi_2), x)
|
|
end
|
|
|
|
def test_strlen()
|
|
cfunc = CFunc.new(@libc['strlen'], TYPE_INT, 'strlen')
|
|
x = cfunc.call(["abc"].pack("p").unpack("l!*"))
|
|
assert_equal("abc".size, x)
|
|
end
|
|
|
|
def test_strcpy()
|
|
buff = "xxxx"
|
|
str = "abc"
|
|
cfunc = CFunc.new(@libc['strcpy'], TYPE_VOIDP, 'strcpy')
|
|
x = cfunc.call(ptr2num(buff,str))
|
|
assert_equal("abc\0", buff)
|
|
assert_equal("abc\0", CPtr.new(x).to_s(4))
|
|
|
|
buff = "xxxx"
|
|
str = "abc"
|
|
cfunc = CFunc.new(@libc['strncpy'], TYPE_VOIDP, 'strncpy')
|
|
x = cfunc.call(ptr2num(buff,str) + [3])
|
|
assert_equal("abcx", buff)
|
|
assert_equal("abcx", CPtr.new(x).to_s(4))
|
|
|
|
ptr = CPtr.malloc(4)
|
|
str = "abc"
|
|
cfunc = CFunc.new(@libc['strcpy'], TYPE_VOIDP, 'strcpy')
|
|
x = cfunc.call([ptr.to_i, *ptr2num(str)])
|
|
assert_equal("abc\0", ptr[0,4])
|
|
assert_equal("abc\0", CPtr.new(x).to_s(4))
|
|
end
|
|
|
|
def test_callback()
|
|
buff = "foobarbaz"
|
|
cb = set_callback(TYPE_INT,2){|x,y| CPtr.new(x)[0] <=> CPtr.new(y)[0]}
|
|
cfunc = CFunc.new(@libc['qsort'], TYPE_VOID, 'qsort')
|
|
cfunc.call(ptr2num(buff) + [buff.size, 1, cb])
|
|
assert_equal('aabbfoorz', buff)
|
|
end
|
|
|
|
def test_dlwrap()
|
|
ary = [0,1,2,4,5]
|
|
addr = dlwrap(ary)
|
|
ary2 = dlunwrap(addr)
|
|
assert_equal(ary, ary2)
|
|
end
|
|
|
|
def test_type_size_t
|
|
assert_equal(DL::TYPE_SSIZE_T, DL::TYPE_SIZE_T.abs)
|
|
end
|
|
|
|
def test_type_uintptr_t
|
|
assert_equal(-DL::TYPE_INTPTR_T, DL::TYPE_UINTPTR_T)
|
|
end
|
|
|
|
def test_sizeof_uintptr_t
|
|
assert_equal(DL::SIZEOF_VOIDP, DL::SIZEOF_INTPTR_T)
|
|
end
|
|
end
|
|
end # module DL
|