mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
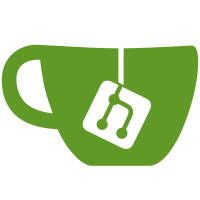
is now parsed in the Encoding it is in without need for translation. * lib/csv/csv.rb: Improved inspect() messages for better IRb support. * lib/csv/csv.rb: Fixed header writing bug reported by Dov Murik. * lib/csv/csv.rb: Use custom separators in parsing header Strings as suggested by Shmulik Regev. * lib/csv/csv.rb: Added a :write_headers option for outputting headers. * lib/csv/csv.rb: Handle open() calls in binary mode whenever we can to workaround a Windows issue where line-ending translation can cause an off-by-one error in seeking back to a non-zero starting position after auto-discovery for :row_sep as suggested by Robert Battle. * lib/csv/csv.rb: Improved the parser to fail faster when fed some forms of invalid CSV that can be detected without reading ahead. * lib/csv/csv.rb: Added a :field_size_limit option to control CSV's lookahead and prevent the parser from biting off more data than it can chew. * lib/csv/csv.rb: Added readers for CSV attributes: col_sep(), row_sep(), quote_char(), field_size_limit(), converters(), unconverted_fields?(), headers(), return_headers?(), write_headers?(), header_converters(), skip_blanks?(), and force_quotes?(). * lib/csv/csv.rb: Cleaned up code syntax to be more inline with Ruby 1.9 than 1.8. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@19441 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
156 lines
3.5 KiB
Ruby
156 lines
3.5 KiB
Ruby
#!/usr/bin/env ruby -w
|
|
# encoding: UTF-8
|
|
|
|
# tc_serialization.rb
|
|
#
|
|
# Created by James Edward Gray II on 2005-10-31.
|
|
# Copyright 2005 James Edward Gray II. You can redistribute or modify this code
|
|
# under the terms of Ruby's license.
|
|
|
|
require "test/unit"
|
|
|
|
require "csv"
|
|
|
|
# An example of how to provide custom CSV serialization.
|
|
class Hash
|
|
def self.csv_load( meta, headers, fields )
|
|
self[*headers.zip(fields).to_a.flatten.map { |e| eval(e) }]
|
|
end
|
|
|
|
def csv_headers
|
|
keys.map { |key| key.inspect }
|
|
end
|
|
|
|
def csv_dump( headers )
|
|
headers.map { |header| fetch(eval(header)).inspect }
|
|
end
|
|
end
|
|
|
|
class TestSerialization < Test::Unit::TestCase
|
|
|
|
### Classes Used to Test Serialization ###
|
|
|
|
class ReadOnlyName
|
|
def initialize( first, last )
|
|
@first, @last = first, last
|
|
end
|
|
|
|
attr_reader :first, :last
|
|
|
|
def ==( other )
|
|
%w{first last}.all? { |att| send(att) == other.send(att) }
|
|
end
|
|
end
|
|
|
|
Name = Struct.new(:first, :last)
|
|
|
|
class FullName < Name
|
|
def initialize( first, last, suffix = nil )
|
|
super(first, last)
|
|
|
|
@suffix = suffix
|
|
end
|
|
|
|
attr_accessor :suffix
|
|
|
|
def ==( other )
|
|
%w{first last suffix}.all? { |att| send(att) == other.send(att) }
|
|
end
|
|
end
|
|
|
|
### Tests ###
|
|
|
|
def test_class_dump
|
|
@names = [ %w{James Gray},
|
|
%w{Dana Gray},
|
|
%w{Greg Brown} ].map do |first, last|
|
|
ReadOnlyName.new(first, last)
|
|
end
|
|
|
|
assert_nothing_raised(Exception) do
|
|
@data = CSV.dump(@names)
|
|
end
|
|
assert_equal(<<-END_CLASS_DUMP.gsub(/^\s*/, ""), @data)
|
|
class,TestSerialization::ReadOnlyName
|
|
@first,@last
|
|
James,Gray
|
|
Dana,Gray
|
|
Greg,Brown
|
|
END_CLASS_DUMP
|
|
end
|
|
|
|
def test_struct_dump
|
|
@names = [ %w{James Gray},
|
|
%w{Dana Gray},
|
|
%w{Greg Brown} ].map do |first, last|
|
|
Name.new(first, last)
|
|
end
|
|
|
|
assert_nothing_raised(Exception) do
|
|
@data = CSV.dump(@names)
|
|
end
|
|
assert_equal(<<-END_STRUCT_DUMP.gsub(/^\s*/, ""), @data)
|
|
class,TestSerialization::Name
|
|
first=,last=
|
|
James,Gray
|
|
Dana,Gray
|
|
Greg,Brown
|
|
END_STRUCT_DUMP
|
|
end
|
|
|
|
def test_inherited_struct_dump
|
|
@names = [ %w{James Gray II},
|
|
%w{Dana Gray},
|
|
%w{Greg Brown} ].map do |first, last, suffix|
|
|
FullName.new(first, last, suffix)
|
|
end
|
|
|
|
assert_nothing_raised(Exception) do
|
|
@data = CSV.dump(@names)
|
|
end
|
|
assert_equal(<<-END_STRUCT_DUMP.gsub(/^\s*/, ""), @data)
|
|
class,TestSerialization::FullName
|
|
@suffix,first=,last=
|
|
II,James,Gray
|
|
,Dana,Gray
|
|
,Greg,Brown
|
|
END_STRUCT_DUMP
|
|
end
|
|
|
|
def test_load
|
|
%w{ test_class_dump
|
|
test_struct_dump
|
|
test_inherited_struct_dump }.each do |test|
|
|
send(test)
|
|
CSV.load(@data).each do |loaded|
|
|
assert_instance_of(@names.first.class, loaded)
|
|
assert_equal(@names.shift, loaded)
|
|
end
|
|
end
|
|
end
|
|
|
|
def test_io
|
|
test_class_dump
|
|
|
|
data_file = File.join(File.dirname(__FILE__), "temp_test_data.csv")
|
|
CSV.dump(@names, File.open(data_file, "w"))
|
|
|
|
assert(File.exist?(data_file))
|
|
assert_equal(<<-END_IO_DUMP.gsub(/^\s*/, ""), File.read(data_file))
|
|
class,TestSerialization::ReadOnlyName
|
|
@first,@last
|
|
James,Gray
|
|
Dana,Gray
|
|
Greg,Brown
|
|
END_IO_DUMP
|
|
|
|
assert_equal(@names, CSV.load(File.open(data_file)))
|
|
|
|
File.unlink(data_file)
|
|
end
|
|
|
|
def test_custom_dump_and_load
|
|
obj = {1 => "simple", :test => Hash}
|
|
assert_equal(obj, CSV.load(CSV.dump([obj])).first)
|
|
end
|
|
end
|