mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
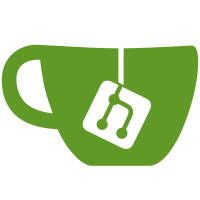
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@19549 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
62 lines
1.6 KiB
Ruby
62 lines
1.6 KiB
Ruby
require 'test/unit'
|
|
require File.join(File.expand_path(File.dirname(__FILE__)), 'gemutilities')
|
|
require File.join(File.expand_path(File.dirname(__FILE__)),
|
|
'gem_installer_test_case')
|
|
require 'rubygems/commands/uninstall_command'
|
|
|
|
class TestGemCommandsUninstallCommand < GemInstallerTestCase
|
|
|
|
def setup
|
|
super
|
|
|
|
ui = MockGemUi.new
|
|
util_setup_gem ui
|
|
|
|
build_rake_in do
|
|
use_ui ui do
|
|
@installer.install
|
|
end
|
|
end
|
|
|
|
@cmd = Gem::Commands::UninstallCommand.new
|
|
@cmd.options[:executables] = true
|
|
@executable = File.join(@gemhome, 'bin', 'executable')
|
|
end
|
|
|
|
def test_execute_removes_executable
|
|
if win_platform?
|
|
assert_equal true, File.exist?(@executable)
|
|
else
|
|
assert_equal true, File.symlink?(@executable)
|
|
end
|
|
|
|
# Evil hack to prevent false removal success
|
|
FileUtils.rm_f @executable
|
|
File.open(@executable, "wb+") {|f| f.puts "binary"}
|
|
|
|
@cmd.options[:args] = Array(@spec.name)
|
|
use_ui @ui do
|
|
@cmd.execute
|
|
end
|
|
|
|
output = @ui.output.split "\n"
|
|
assert_match(/Removing executable/, output.shift)
|
|
assert_match(/Successfully uninstalled/, output.shift)
|
|
assert_equal false, File.exist?(@executable)
|
|
assert_nil output.shift, "UI output should have contained only two lines"
|
|
end
|
|
|
|
def test_execute_not_installed
|
|
@cmd.options[:args] = ["foo"]
|
|
e = assert_raise(Gem::InstallError) do
|
|
use_ui @ui do
|
|
@cmd.execute
|
|
end
|
|
end
|
|
|
|
assert_match(/\AUnknown gem foo >= 0$/, e.message)
|
|
output = @ui.output.split "\n"
|
|
assert output.empty?, "UI output should be empty after an uninstall error"
|
|
end
|
|
end
|
|
|