mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
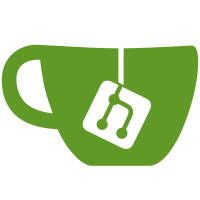
* lib/irb.rb (IRB::Irb#eval_input): update `_` after exception. [ruby-core:86989] [Bug #14749] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@63409 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
76 lines
1.9 KiB
Ruby
76 lines
1.9 KiB
Ruby
# frozen_string_literal: false
|
|
require 'test/unit'
|
|
require 'tempfile'
|
|
require 'irb'
|
|
require 'rubygems' if defined?(Gem)
|
|
|
|
module TestIRB
|
|
class TestContext < Test::Unit::TestCase
|
|
class TestInputMethod < ::IRB::InputMethod
|
|
attr_reader :list, :line_no
|
|
|
|
def initialize(list = [])
|
|
super("test")
|
|
@line_no = 0
|
|
@list = list
|
|
end
|
|
|
|
def gets
|
|
@list[@line_no]&.tap {@line_no += 1}
|
|
end
|
|
|
|
def eof?
|
|
@line_no >= @list.size
|
|
end
|
|
|
|
def encoding
|
|
Encoding.default_external
|
|
end
|
|
end
|
|
|
|
def setup
|
|
IRB.init_config(nil)
|
|
IRB.conf[:USE_READLINE] = false
|
|
IRB.conf[:VERBOSE] = false
|
|
workspace = IRB::WorkSpace.new(Object.new)
|
|
@context = IRB::Context.new(nil, workspace, TestInputMethod.new)
|
|
end
|
|
|
|
def test_last_value
|
|
assert_nil(@context.last_value)
|
|
assert_nil(@context.evaluate('_', 1))
|
|
obj = Object.new
|
|
@context.set_last_value(obj)
|
|
assert_same(obj, @context.last_value)
|
|
assert_same(obj, @context.evaluate('_', 1))
|
|
end
|
|
|
|
def test_evaluate_with_exception
|
|
assert_nil(@context.evaluate("$!", 1))
|
|
e = assert_raise_with_message(RuntimeError, 'foo') {
|
|
@context.evaluate("raise 'foo'", 1)
|
|
}
|
|
assert_equal('foo', e.message)
|
|
assert_same(e, @context.evaluate('$!', 1, exception: e))
|
|
end
|
|
|
|
def test_eval_input
|
|
input = TestInputMethod.new([
|
|
"raise 'Foo'\n",
|
|
"_\n",
|
|
"0\n",
|
|
"_\n",
|
|
])
|
|
irb = IRB::Irb.new(IRB::WorkSpace.new(Object.new), input)
|
|
out, err = capture_io do
|
|
irb.eval_input
|
|
end
|
|
assert_empty err
|
|
assert_pattern_list([:*, /RuntimeError \(.*Foo.*\).*\n/,
|
|
:*, /#<RuntimeError: Foo>\n/,
|
|
:*, /0$/,
|
|
:*, /0$/,
|
|
/\s*/], out)
|
|
end
|
|
end
|
|
end
|