mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
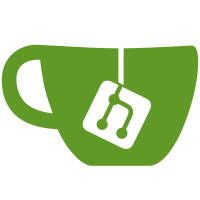
This allows easy differentiation between ABI incompatible platforms like MSWIN64 and MSVCRT-based MINGW32. This also implicates a distinct rubygem platform which is also "x64-mingw-ucrt". Although the term "mingw32" is the OS-part for 64 bit systems as well, the "32" is misleading and confusing for many users. Therefore the new platform string drops the "32" from the OS part to just "mingw". This conforms to the common practice of windows platform testing per RUBY_PLATFORM=~/mswin|mingw/ .
216 lines
6.7 KiB
Ruby
216 lines
6.7 KiB
Ruby
# frozen_string_literal: true
|
|
require_relative "deprecate"
|
|
|
|
##
|
|
# Available list of platforms for targeting Gem installations.
|
|
#
|
|
# See `gem help platform` for information on platform matching.
|
|
|
|
class Gem::Platform
|
|
@local = nil
|
|
|
|
attr_accessor :cpu, :os, :version
|
|
|
|
def self.local
|
|
arch = RbConfig::CONFIG['arch']
|
|
arch = "#{arch}_60" if arch =~ /mswin(?:32|64)$/
|
|
@local ||= new(arch)
|
|
end
|
|
|
|
def self.match(platform)
|
|
match_platforms?(platform, Gem.platforms)
|
|
end
|
|
|
|
def self.match_platforms?(platform, platforms)
|
|
platforms.any? do |local_platform|
|
|
platform.nil? or
|
|
local_platform == platform or
|
|
(local_platform != Gem::Platform::RUBY and local_platform =~ platform)
|
|
end
|
|
end
|
|
private_class_method :match_platforms?
|
|
|
|
def self.match_spec?(spec)
|
|
match_gem?(spec.platform, spec.name)
|
|
end
|
|
|
|
def self.match_gem?(platform, gem_name)
|
|
# Note: this method might be redefined by Ruby implementations to
|
|
# customize behavior per RUBY_ENGINE, gem_name or other criteria.
|
|
match_platforms?(platform, Gem.platforms)
|
|
end
|
|
|
|
def self.sort_priority(platform)
|
|
platform == Gem::Platform::RUBY ? -1 : 1
|
|
end
|
|
|
|
def self.installable?(spec)
|
|
if spec.respond_to? :installable_platform?
|
|
spec.installable_platform?
|
|
else
|
|
match_spec? spec
|
|
end
|
|
end
|
|
|
|
def self.new(arch) # :nodoc:
|
|
case arch
|
|
when Gem::Platform::CURRENT then
|
|
Gem::Platform.local
|
|
when Gem::Platform::RUBY, nil, '' then
|
|
Gem::Platform::RUBY
|
|
else
|
|
super
|
|
end
|
|
end
|
|
|
|
def initialize(arch)
|
|
case arch
|
|
when Array then
|
|
@cpu, @os, @version = arch
|
|
when String then
|
|
arch = arch.split '-'
|
|
|
|
if arch.length > 2 and arch.last !~ /\d/ # reassemble x86-linux-gnu
|
|
extra = arch.pop
|
|
arch.last << "-#{extra}"
|
|
end
|
|
|
|
cpu = arch.shift
|
|
|
|
@cpu = case cpu
|
|
when /i\d86/ then 'x86'
|
|
else cpu
|
|
end
|
|
|
|
if arch.length == 2 and arch.last =~ /^\d+(\.\d+)?$/ # for command-line
|
|
@os, @version = arch
|
|
return
|
|
end
|
|
|
|
os, = arch
|
|
@cpu, os = nil, cpu if os.nil? # legacy jruby
|
|
|
|
@os, @version = case os
|
|
when /aix(\d+)?/ then [ 'aix', $1 ]
|
|
when /cygwin/ then [ 'cygwin', nil ]
|
|
when /darwin(\d+)?/ then [ 'darwin', $1 ]
|
|
when /^macruby$/ then [ 'macruby', nil ]
|
|
when /freebsd(\d+)?/ then [ 'freebsd', $1 ]
|
|
when /hpux(\d+)?/ then [ 'hpux', $1 ]
|
|
when /^java$/, /^jruby$/ then [ 'java', nil ]
|
|
when /^java([\d.]*)/ then [ 'java', $1 ]
|
|
when /^dalvik(\d+)?$/ then [ 'dalvik', $1 ]
|
|
when /^dotnet$/ then [ 'dotnet', nil ]
|
|
when /^dotnet([\d.]*)/ then [ 'dotnet', $1 ]
|
|
when /linux-?((?!gnu)\w+)?/ then [ 'linux', $1 ]
|
|
when /mingw32/ then [ 'mingw32', nil ]
|
|
when /mingw-?(\w+)?/ then [ 'mingw', $1 ]
|
|
when /(mswin\d+)(\_(\d+))?/ then
|
|
os, version = $1, $3
|
|
@cpu = 'x86' if @cpu.nil? and os =~ /32$/
|
|
[os, version]
|
|
when /netbsdelf/ then [ 'netbsdelf', nil ]
|
|
when /openbsd(\d+\.\d+)?/ then [ 'openbsd', $1 ]
|
|
when /bitrig(\d+\.\d+)?/ then [ 'bitrig', $1 ]
|
|
when /solaris(\d+\.\d+)?/ then [ 'solaris', $1 ]
|
|
# test
|
|
when /^(\w+_platform)(\d+)?/ then [ $1, $2 ]
|
|
else [ 'unknown', nil ]
|
|
end
|
|
when Gem::Platform then
|
|
@cpu = arch.cpu
|
|
@os = arch.os
|
|
@version = arch.version
|
|
else
|
|
raise ArgumentError, "invalid argument #{arch.inspect}"
|
|
end
|
|
end
|
|
|
|
def to_a
|
|
[@cpu, @os, @version]
|
|
end
|
|
|
|
def to_s
|
|
to_a.compact.join '-'
|
|
end
|
|
|
|
##
|
|
# Is +other+ equal to this platform? Two platforms are equal if they have
|
|
# the same CPU, OS and version.
|
|
|
|
def ==(other)
|
|
self.class === other and to_a == other.to_a
|
|
end
|
|
|
|
alias :eql? :==
|
|
|
|
def hash # :nodoc:
|
|
to_a.hash
|
|
end
|
|
|
|
##
|
|
# Does +other+ match this platform? Two platforms match if they have the
|
|
# same CPU, or either has a CPU of 'universal', they have the same OS, and
|
|
# they have the same version, or either has no version.
|
|
#
|
|
# Additionally, the platform will match if the local CPU is 'arm' and the
|
|
# other CPU starts with "arm" (for generic ARM family support).
|
|
|
|
def ===(other)
|
|
return nil unless Gem::Platform === other
|
|
|
|
# cpu
|
|
([nil,'universal'].include?(@cpu) or [nil, 'universal'].include?(other.cpu) or @cpu == other.cpu or
|
|
(@cpu == 'arm' and other.cpu.start_with?("arm"))) and
|
|
|
|
# os
|
|
@os == other.os and
|
|
|
|
# version
|
|
(@version.nil? or other.version.nil? or @version == other.version)
|
|
end
|
|
|
|
##
|
|
# Does +other+ match this platform? If +other+ is a String it will be
|
|
# converted to a Gem::Platform first. See #=== for matching rules.
|
|
|
|
def =~(other)
|
|
case other
|
|
when Gem::Platform then # nop
|
|
when String then
|
|
# This data is from http://gems.rubyforge.org/gems/yaml on 19 Aug 2007
|
|
other = case other
|
|
when /^i686-darwin(\d)/ then ['x86', 'darwin', $1 ]
|
|
when /^i\d86-linux/ then ['x86', 'linux', nil ]
|
|
when 'java', 'jruby' then [nil, 'java', nil ]
|
|
when /^dalvik(\d+)?$/ then [nil, 'dalvik', $1 ]
|
|
when /dotnet(\-(\d+\.\d+))?/ then ['universal','dotnet', $2 ]
|
|
when /mswin32(\_(\d+))?/ then ['x86', 'mswin32', $2 ]
|
|
when /mswin64(\_(\d+))?/ then ['x64', 'mswin64', $2 ]
|
|
when 'powerpc-darwin' then ['powerpc', 'darwin', nil ]
|
|
when /powerpc-darwin(\d)/ then ['powerpc', 'darwin', $1 ]
|
|
when /sparc-solaris2.8/ then ['sparc', 'solaris', '2.8' ]
|
|
when /universal-darwin(\d)/ then ['universal', 'darwin', $1 ]
|
|
else other
|
|
end
|
|
|
|
other = Gem::Platform.new other
|
|
else
|
|
return nil
|
|
end
|
|
|
|
self === other
|
|
end
|
|
|
|
##
|
|
# A pure-Ruby gem that may use Gem::Specification#extensions to build
|
|
# binary files.
|
|
|
|
RUBY = 'ruby'.freeze
|
|
|
|
##
|
|
# A platform-specific gem that is built for the packaging Ruby's platform.
|
|
# This will be replaced with Gem::Platform::local.
|
|
|
|
CURRENT = 'current'.freeze
|
|
end
|