mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
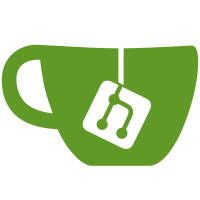
Compatibly renamed Gem::DependencyResolver to Gem::Resolver. Added support for git gems in gem.deps.rb and Gemfile. Fixed resolver bugs. * test/rubygems: ditto. * lib/rubygems/LICENSE.txt: Updated to license from RubyGems trunk. [ruby-trunk - Bug #9086] * lib/rubygems/commands/which_command.rb: RubyGems now indicates failure when any file is missing. [ruby-trunk - Bug #9004] * lib/rubygems/ext/builder: Extensions are now installed into the extension install directory and the first directory in the require path from the gem. This allows backwards compatibility with msgpack and other gems that calculate full require paths. [ruby-trunk - Bug #9106] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@43714 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
149 lines
2.5 KiB
Ruby
149 lines
2.5 KiB
Ruby
require 'rubygems/source'
|
|
|
|
##
|
|
# The SourceList represents the sources rubygems has been configured to use.
|
|
# A source may be created from an array of sources:
|
|
#
|
|
# Gem::SourceList.from %w[https://rubygems.example https://internal.example]
|
|
#
|
|
# Or by adding them:
|
|
#
|
|
# sources = Gem::SourceList.new
|
|
# sources.add 'https://rubygems.example'
|
|
#
|
|
# The most common way to get a SourceList is Gem.sources.
|
|
|
|
class Gem::SourceList
|
|
|
|
include Enumerable
|
|
|
|
##
|
|
# Creates a new SourceList
|
|
|
|
def initialize
|
|
@sources = []
|
|
end
|
|
|
|
##
|
|
# The sources in this list
|
|
|
|
attr_reader :sources
|
|
|
|
##
|
|
# Creates a new SourceList from an array of sources.
|
|
|
|
def self.from(ary)
|
|
list = new
|
|
|
|
list.replace ary
|
|
|
|
return list
|
|
end
|
|
|
|
def initialize_copy(other) # :nodoc:
|
|
@sources = @sources.dup
|
|
end
|
|
|
|
##
|
|
# Appends +obj+ to the source list which may be a Gem::Source, URI or URI
|
|
# String.
|
|
|
|
def <<(obj)
|
|
src = case obj
|
|
when URI
|
|
Gem::Source.new(obj)
|
|
when Gem::Source
|
|
obj
|
|
else
|
|
Gem::Source.new(URI.parse(obj))
|
|
end
|
|
|
|
@sources << src
|
|
src
|
|
end
|
|
|
|
##
|
|
# Replaces this SourceList with the sources in +other+ See #<< for
|
|
# acceptable items in +other+.
|
|
|
|
def replace(other)
|
|
clear
|
|
|
|
other.each do |x|
|
|
self << x
|
|
end
|
|
|
|
self
|
|
end
|
|
|
|
##
|
|
# Removes all sources from the SourceList.
|
|
|
|
def clear
|
|
@sources.clear
|
|
end
|
|
|
|
##
|
|
# Yields each source URI in the list.
|
|
|
|
def each
|
|
@sources.each { |s| yield s.uri.to_s }
|
|
end
|
|
|
|
##
|
|
# Yields each source in the list.
|
|
|
|
def each_source(&b)
|
|
@sources.each(&b)
|
|
end
|
|
|
|
##
|
|
# Returns true if there are no sources in this SourceList.
|
|
|
|
def empty?
|
|
@sources.empty?
|
|
end
|
|
|
|
def == other # :nodoc:
|
|
to_a == other
|
|
end
|
|
|
|
##
|
|
# Returns an Array of source URI Strings.
|
|
|
|
def to_a
|
|
@sources.map { |x| x.uri.to_s }
|
|
end
|
|
|
|
alias_method :to_ary, :to_a
|
|
|
|
##
|
|
# Returns the first source in the list.
|
|
|
|
def first
|
|
@sources.first
|
|
end
|
|
|
|
##
|
|
# Returns true if this source list includes +other+ which may be a
|
|
# Gem::Source or a source URI.
|
|
|
|
def include?(other)
|
|
if other.kind_of? Gem::Source
|
|
@sources.include? other
|
|
else
|
|
@sources.find { |x| x.uri.to_s == other.to_s }
|
|
end
|
|
end
|
|
|
|
##
|
|
# Deletes +source+ from the source list which may be a Gem::Source or a URI.
|
|
|
|
def delete source
|
|
if source.kind_of? Gem::Source
|
|
@sources.delete source
|
|
else
|
|
@sources.delete_if { |x| x.uri.to_s == source.to_s }
|
|
end
|
|
end
|
|
end
|