mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
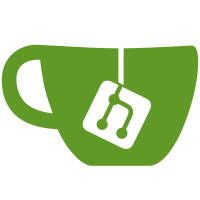
* parse.y (parser_yylex): warn ambiguous parentheses after a space in method definitions. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@56927 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
61 lines
1,004 B
Ruby
61 lines
1,004 B
Ruby
# frozen_string_literal: false
|
|
# date.rb: Written by Tadayoshi Funaba 1998-2011
|
|
|
|
require 'date_core'
|
|
|
|
class Date
|
|
|
|
class Infinity < Numeric # :nodoc:
|
|
|
|
include Comparable
|
|
|
|
def initialize(d=1) @d = d <=> 0 end
|
|
|
|
def d() @d end
|
|
|
|
protected :d
|
|
|
|
def zero?() false end
|
|
def finite?() false end
|
|
def infinite?() d.nonzero? end
|
|
def nan?() d.zero? end
|
|
|
|
def abs() self.class.new end
|
|
|
|
def -@() self.class.new(-d) end
|
|
def +@() self.class.new(+d) end
|
|
|
|
def <=>(other)
|
|
case other
|
|
when Infinity; return d <=> other.d
|
|
when Numeric; return d
|
|
else
|
|
begin
|
|
l, r = other.coerce(self)
|
|
return l <=> r
|
|
rescue NoMethodError
|
|
end
|
|
end
|
|
nil
|
|
end
|
|
|
|
def coerce(other)
|
|
case other
|
|
when Numeric; return -d, d
|
|
else
|
|
super
|
|
end
|
|
end
|
|
|
|
def to_f
|
|
return 0 if @d == 0
|
|
if @d > 0
|
|
Float::INFINITY
|
|
else
|
|
-Float::INFINITY
|
|
end
|
|
end
|
|
|
|
end
|
|
|
|
end
|