mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
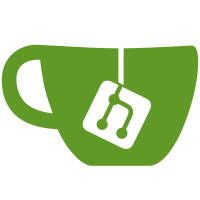
Compatibly renamed Gem::DependencyResolver to Gem::Resolver. Added support for git gems in gem.deps.rb and Gemfile. Fixed resolver bugs. * test/rubygems: ditto. * lib/rubygems/LICENSE.txt: Updated to license from RubyGems trunk. [ruby-trunk - Bug #9086] * lib/rubygems/commands/which_command.rb: RubyGems now indicates failure when any file is missing. [ruby-trunk - Bug #9004] * lib/rubygems/ext/builder: Extensions are now installed into the extension install directory and the first directory in the require path from the gem. This allows backwards compatibility with msgpack and other gems that calculate full require paths. [ruby-trunk - Bug #9106] git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@43714 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
128 lines
2.6 KiB
Ruby
128 lines
2.6 KiB
Ruby
##
|
|
# The local source finds gems in the current directory for fulfilling
|
|
# dependencies.
|
|
|
|
class Gem::Source::Local < Gem::Source
|
|
|
|
def initialize # :nodoc:
|
|
@specs = nil
|
|
@api_uri = nil
|
|
@uri = nil
|
|
end
|
|
|
|
##
|
|
# Local sorts before Gem::Source and after Gem::Source::Installed
|
|
|
|
def <=> other
|
|
case other
|
|
when Gem::Source::Installed then
|
|
-1
|
|
when Gem::Source::Local then
|
|
0
|
|
when Gem::Source then
|
|
1
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
|
|
def inspect # :nodoc:
|
|
keys = @specs ? @specs.keys.sort : 'NOT LOADED'
|
|
"#<%s specs: %p>" % [self.class, keys]
|
|
end
|
|
|
|
def load_specs type # :nodoc:
|
|
names = []
|
|
|
|
@specs = {}
|
|
|
|
Dir["*.gem"].each do |file|
|
|
begin
|
|
pkg = Gem::Package.new(file)
|
|
rescue SystemCallError, Gem::Package::FormatError
|
|
# ignore
|
|
else
|
|
tup = pkg.spec.name_tuple
|
|
@specs[tup] = [File.expand_path(file), pkg]
|
|
|
|
case type
|
|
when :released
|
|
unless pkg.spec.version.prerelease?
|
|
names << pkg.spec.name_tuple
|
|
end
|
|
when :prerelease
|
|
if pkg.spec.version.prerelease?
|
|
names << pkg.spec.name_tuple
|
|
end
|
|
when :latest
|
|
tup = pkg.spec.name_tuple
|
|
|
|
cur = names.find { |x| x.name == tup.name }
|
|
if !cur
|
|
names << tup
|
|
elsif cur.version < tup.version
|
|
names.delete cur
|
|
names << tup
|
|
end
|
|
else
|
|
names << pkg.spec.name_tuple
|
|
end
|
|
end
|
|
end
|
|
|
|
names
|
|
end
|
|
|
|
def find_gem gem_name, version = Gem::Requirement.default, # :nodoc:
|
|
prerelease = false
|
|
load_specs :complete
|
|
|
|
found = []
|
|
|
|
@specs.each do |n, data|
|
|
if n.name == gem_name
|
|
s = data[1].spec
|
|
|
|
if version.satisfied_by?(s.version)
|
|
if prerelease
|
|
found << s
|
|
elsif !s.version.prerelease?
|
|
found << s
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
found.max_by { |s| s.version }
|
|
end
|
|
|
|
def fetch_spec name # :nodoc:
|
|
load_specs :complete
|
|
|
|
if data = @specs[name]
|
|
data.last.spec
|
|
else
|
|
raise Gem::Exception, "Unable to find spec for #{name.inspect}"
|
|
end
|
|
end
|
|
|
|
def download spec, cache_dir = nil # :nodoc:
|
|
load_specs :complete
|
|
|
|
@specs.each do |name, data|
|
|
return data[0] if data[1].spec == spec
|
|
end
|
|
|
|
raise Gem::Exception, "Unable to find file for '#{spec.full_name}'"
|
|
end
|
|
|
|
def pretty_print q # :nodoc:
|
|
q.group 2, '[Local gems:', ']' do
|
|
q.breakable
|
|
q.seplist @specs.keys do |v|
|
|
q.text v.full_name
|
|
end
|
|
end
|
|
end
|
|
|
|
end
|