mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
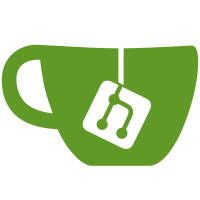
Add a new macro BASE_SLOT_SIZE that determines the slot size. For Variable Width Allocation (compiled with USE_RVARGC=1), all slot sizes are powers-of-2 multiples of BASE_SLOT_SIZE. For USE_RVARGC=0, BASE_SLOT_SIZE is set to sizeof(RVALUE).
37 lines
965 B
Ruby
37 lines
965 B
Ruby
# frozen_string_literal: false
|
|
require 'test/unit'
|
|
require "-test-/string"
|
|
|
|
class Test_StrSetLen < Test::Unit::TestCase
|
|
def setup
|
|
# Make string long enough so that it is not embedded
|
|
@range_end = ("0".ord + GC::INTERNAL_CONSTANTS[:BASE_SLOT_SIZE]).chr
|
|
@s0 = [*"0"..@range_end].join("").freeze
|
|
@s1 = Bug::String.new(@s0)
|
|
end
|
|
|
|
def teardown
|
|
orig = [*"0"..@range_end].join("")
|
|
assert_equal(orig, @s0)
|
|
end
|
|
|
|
def test_non_shared
|
|
@s1.modify!
|
|
assert_equal("012", @s1.set_len(3))
|
|
end
|
|
|
|
def test_shared
|
|
assert_raise(RuntimeError) {
|
|
@s1.set_len(3)
|
|
}
|
|
end
|
|
|
|
def test_capacity_equals_to_new_size
|
|
bug12757 = "[ruby-core:77257] [Bug #12757]"
|
|
# fill to ensure capacity does not decrease with force_encoding
|
|
str = Bug::String.new("\x00" * 128, capacity: 128)
|
|
str.force_encoding("UTF-32BE")
|
|
assert_equal 128, Bug::String.capacity(str)
|
|
assert_equal 127, str.set_len(127).bytesize, bug12757
|
|
end
|
|
end
|