mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
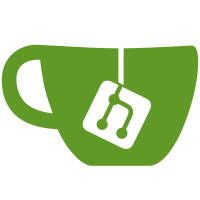
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@61504 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
68 lines
943 B
Ruby
68 lines
943 B
Ruby
module RangeSpecs
|
|
class TenfoldSucc
|
|
include Comparable
|
|
|
|
attr_reader :n
|
|
|
|
def initialize(n)
|
|
@n = n
|
|
end
|
|
|
|
def <=>(other)
|
|
@n <=> other.n
|
|
end
|
|
|
|
def succ
|
|
self.class.new(@n * 10)
|
|
end
|
|
end
|
|
|
|
# Custom Range classes Xs and Ys
|
|
class Custom
|
|
include Comparable
|
|
attr_reader :length
|
|
|
|
def initialize(n)
|
|
@length = n
|
|
end
|
|
|
|
def eql?(other)
|
|
inspect.eql? other.inspect
|
|
end
|
|
alias :== :eql?
|
|
|
|
def inspect
|
|
'custom'
|
|
end
|
|
|
|
def <=>(other)
|
|
@length <=> other.length
|
|
end
|
|
end
|
|
|
|
class Xs < Custom # represent a string of 'x's
|
|
def succ
|
|
Xs.new(@length + 1)
|
|
end
|
|
|
|
def inspect
|
|
'x' * @length
|
|
end
|
|
end
|
|
|
|
class Ys < Custom # represent a string of 'y's
|
|
def succ
|
|
Ys.new(@length + 1)
|
|
end
|
|
|
|
def inspect
|
|
'y' * @length
|
|
end
|
|
end
|
|
|
|
class MyRange < Range
|
|
end
|
|
|
|
class ComparisonError < RuntimeError
|
|
end
|
|
end
|