mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
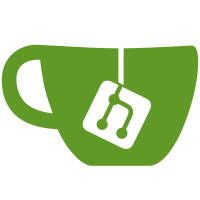
git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@19547 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
63 lines
873 B
Ruby
63 lines
873 B
Ruby
#!/usr/bin/env ruby
|
|
#--
|
|
# Copyright 2006 by Chad Fowler, Rich Kilmer, Jim Weirich and others.
|
|
# All rights reserved.
|
|
# See LICENSE.txt for permissions.
|
|
#++
|
|
|
|
|
|
require 'stringio'
|
|
require 'rubygems/user_interaction'
|
|
|
|
class MockGemUi < Gem::StreamUI
|
|
class TermError < RuntimeError; end
|
|
|
|
module TTY
|
|
|
|
attr_accessor :tty
|
|
|
|
def tty?()
|
|
@tty = true unless defined?(@tty)
|
|
@tty
|
|
end
|
|
|
|
end
|
|
|
|
def initialize(input = "")
|
|
ins = StringIO.new input
|
|
outs = StringIO.new
|
|
errs = StringIO.new
|
|
|
|
ins.extend TTY
|
|
outs.extend TTY
|
|
errs.extend TTY
|
|
|
|
super ins, outs, errs
|
|
|
|
@terminated = false
|
|
end
|
|
|
|
def input
|
|
@ins.string
|
|
end
|
|
|
|
def output
|
|
@outs.string
|
|
end
|
|
|
|
def error
|
|
@errs.string
|
|
end
|
|
|
|
def terminated?
|
|
@terminated
|
|
end
|
|
|
|
def terminate_interaction(status=0)
|
|
@terminated = true
|
|
|
|
raise TermError
|
|
end
|
|
|
|
end
|
|
|